Article Continues Under
Raphaël is a lightweight JavaScript library that renders dynamic SVG graphics together with charts, graphs, vector-based animations and GUI parts proper into your internet pages. Now, you’re most likely pondering, I can already do that with jQuery, Google Charts, and even Flash! Whereas that is true, Raphaël reveals new potentialities not at present obtainable with these different applied sciences. Let’s learn to create inline scalable vector photos that work throughout browsers and degrade gracefully.
Open language for an open internet#section2
The net is all about open requirements and unencumbered applied sciences. Raphaël, which makes use of W3C commonplace SVG and an open supply MIT License, matches the open internet completely.
However, what about Web Explorer 6? It doesn’t assist SVG! That’s true, however IE6 does assist its personal VML (Vector Markup Language). (IE9 is slated to assist SVG 1.1. Word that Raphaël depends on function detection relatively than consumer agent (UA) sniffing. IE7 and IE8 don’t know what to do with an SVG file, so Raphaël robotically switches modes for you.) Raphaël abstracts all that browser nonsense away so that you simply don’t should cope with it. The identical JavaScript code will create lovely SVG or VML primarily based on the browser’s capabilities. Write it as soon as and it runs anyplace. By sidestepping Flash for vector graphics, you make your output obtainable on extra gadgets, together with cellular WebKit browsers and iOS gadgets. Your fancy, interactive, scalable graphics will look lovely at any decision on any supported gadget.
What about jQuery? There are many graphics plugins. Why ought to I swap to Raphaël?
There are many fascinating jQuery graphics plugins, corresponding to jqPlot, Flot, jQuery SVG, and others. With jQuery, you decide to 50+ KB for the minified library. Then, add the UI core, some plugins, and the additional libraries to get it working for IE. Byte for byte, jQuery packs a punch, however you get further bits you don’t at all times want.
Raphaël is smaller total for creating primary vector graphics. Watching your KB weight is one thing to contemplate when growing giant scale purposes, however so is utilizing the most effective software for the job.
It doesn’t should be an both/or state of affairs. If you’re already utilizing jQuery, it’s attainable to drop in Raphaël with out conflicts and blend and match the 2. Many of those JavaScript libraries reside in concord with one another, which makes our lives as builders simpler.
Earlier than we had all these libraries, instruments, and internet companies at our disposal, dynamically generated graphics have been created on the server by Frequent Gateway Interface (CGI) scripts. How issues have modified. Now we have now entry to companies corresponding to Google charts and others utilizing Representational State Switch (REST). Simply specify the parameters to get a stunning graphic in return.
Embedding Google charts in an <img>
ingredient is a quick and versatile option to create a pleasant bar chart on the fly, but it surely does have its downsides. As a picture ingredient, you might be left solely with the alt
attribute. You need to use longdesc
or a hacked picture map to label areas. All the info is locked up within the pixels and never within the HTML. Static photos are rendered at a sure dimension. Which means that as you zoom in, the picture turns into pixelated. As you start to construct skilled workflows, you’ll run into issues with rasterized graphics corresponding to GIF, JPEG, and PNG, which don’t scale up or down very effectively. SVG, alternatively, is made from vectors, so it’s decision impartial. Because the internet is accessible in all sizes and styles, and since knowledge is extra steadily transitioned on and off the net, graphics should retain their decision from journal high quality to low-end 72dpi display resolutions and every part in between.
Progressive Enhancement#section6
Raphaël makes use of JavaScript to inject the SVG or VML into the HTML web page. Which means that your browser must have JavaScript assist to see the ensuing graphics. Whilst you’d anticipate most individuals looking your web site in any fashionable browser to have JavaScript turned on in addition to assist SVG, your largest and most essential clients don’t. Search engine bots, corresponding to Google Bot, don’t render JavaScript. It doesn’t “see” your lovely graphs. It sees an empty div
with no content material!
That is the place progressive enhancement is essential. The uncooked HTML knowledge serves as your baseline. It may not look fairly as a desk or checklist, however it’s semantically right and provides hooks for serps to index your content material. It’s now attainable to make use of the HTML as your knowledge supply when developing the Raphaël graphic.
Energy | 45% |
---|---|
Saturated Fat | 25% |
Protein | 15% |
Vitamin B | 10% |
Vitamin C | 5% |
perform pieMe() {
var values = [],
labels = [],
nfTable=doc.getElementById(“nutritionFacts”),
i = 0,
rows = nfTable.rows.size;
for(;i < rows;i++) {
trRow = nfTable.getElementsByTagName(“tr”)[i];
labels.push(trRow.getElementsByTagName(“th”)[0].firstChild.nodeValue);
values.push(parseInt(trRow.getElementsByTagName(“td”)[0].firstChild.nodeValue, 10));
}
nfTable.fashion.place=’absolute’;
nfTable.fashion.left=’-999em’;
Raphael(“myHolderPie”, 540, 400).pieChart(270, 200, 100, values, labels, “#fff”);
}
pieMe();
First we create a desk of values that add as much as 100%. These are the values for every wedge within the pie chart. We are able to improve the common desk if the browser helps it.
<desk id="nutritionFacts">
<caption>Dietary info for product X</caption>
<tr>
<th scope="row">Energy</th>
<td>45%</td>
</tr>
<tr>
<th scope="row">Saturated Fat</th>
<td>25%</td>
</tr>
<tr>
<th scope="row">Protein</th>
<td>15%</td>
</tr>
<tr>
<th scope="row">Vitamin B</th>
<td>10%</td>
</tr>
<tr>
<th scope="row">Vitamin C</th>
<td>5%</td>
</tr>
</desk>
Now that we have now our baseline uncooked knowledge, we are able to add a number of exterior libraries. First we should embrace Raphaël.
<script src="https://alistapart.com/article/svg-with-a-little-help-from-raphael/raphael.js" sort="textual content/javascript" charset="utf-8"></script>
Then we’ll use Raphaël’s plugin system to incorporate the Pie Charts plugin.
<script src="https://alistapart.com/article/svg-with-a-little-help-from-raphael/pie.js" sort="textual content/javascript" charset="utf-8"></script>
This provides us entry to all of the instruments we have to convert the desk into an SVG graphic with ease. To generate the pie chart, we should first create two arrays, one for the labels and a second one for the values.
var values = [], labels = [], nfTable = doc.getElementById("nutritionFacts"), i = 0, rows = nfTable.rows.size;for( ;i < rows; i++) { trRow = nfTable.getElementsByTagName("tr")<i>; labels.push(trRow.getElementsByTagName("th")[0].firstChild.nodeValue); values.push(parseInt(trRow.getElementsByTagName("td")[0] .firstChild.nodeValue, 10)); }nfTable.fashion.place = 'absolute'; nfTable.fashion.left="-999em";
Now, we discover every <tr>
ingredient and loop by means of, pushing every <th>
into the label array and every <td>
into the worth array. Lastly, we cover the desk since we’ll change it with the pie chart later.
raphael("myHolderPie", 540, 400).pieChart(270, 200, 100, values, labels, "#fff");
To render the pie chart, we use the Raphaël object, specify a component id
and a few dimensions. On this case, we’re utilizing the myHolderPie div
. We give it a width of 540 pixels and a peak of 400 pixels. We then connect the pieChart perform to that space. The pieChart perform takes a number of variables, together with the middle level from the left and proper. On this case we’re beginning 270px from the left facet of the holder ingredient. The following argument is 200, which is the variety of pixels from the highest of the holder ingredient. The 100 tells the code to make a pie chart with a diameter of 100 pixels. (Go forward—attempt to improve that quantity to 200 or lower it to 50—see what occurs.) The following two arguments are the values for the pie chart and the labels we constructed utilizing jQuery. The final argument is the HEX worth for the wedge outlines. If you happen to delete this argument, then there received’t be any borders.
gRaphaël has extra details about the forms of graphs that you should utilize to create graphics.
Take into account that each time you substitute a desk or different info with a flowery graphic or interactive interface, you want to take into account what you achieve and what you lose. Accessibility is a crucial consideration. In case your readers solely have a keyboard, can they nonetheless use the tab key to entry the info? Can blind customers entry the knowledge within the new content material as they might with the unique? On this case an accessible desk is changed by a less-accessible asset, however the outdated desk has been hidden and continues to be obtainable to display reader customers. Nevertheless, the brand new content material does restrict use by keyboard customers. Assist for the W3C’s Accessible Wealthy Web Purposes (ARIA) roles and for tabIndex="0"
within the SVG would assist tackle accessibility constraints sooner or later, however these will not be a part of the Raphaël plugin immediately.
Animations#section7
Raphaël additionally has loads of fascinating animation skills constructed into it. One actually fascinating one is the power to hint a path. Think about you’re employed for the Edinburgh Vacationer council. The Edinburgh Citadel is a well-liked vacation spot, and also you need to present the quicket and best option to get there from the prepare station. How cool it will be if you happen to may plot a strolling route!
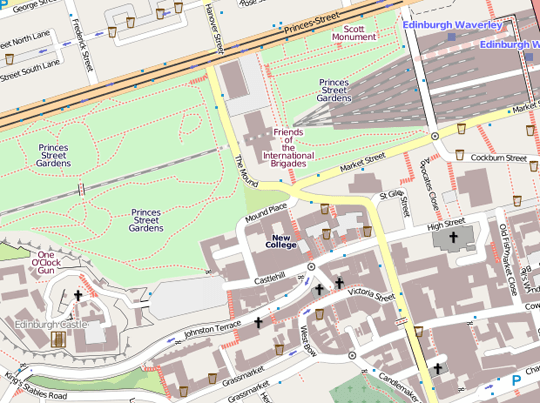
perform tracePath(){
doc.getElementById(“myMapHolder”).removeChild(doc.getElementById(“myMapHolder”).childNodes[0]);
var r = Raphael(“myMapHolder”, 540, 403), c = r.picture(“map.png”, 0, 0, 540, 403), p = r.path(“M513.859,35.222l-95.332,24.667l18.666,76.667l-131.334,46c0,0-10.667,3.333-11.333,13.333c-0.891,13.371-42.748,23.018-42.748,23.018s-13.252,3.648-9.252,18.315s14.667,43.333,14.667,43.333l-104.667,22.667”).attr({stroke: “#f00”, opacity: .7, “stroke-width”: 5}),e = r.ellipse(513.859,35.333, 7, 7).attr({stroke: “none”, opacity: .7, fill: “#f00”});
perform run() {
e.animateAlong(p, 20000, false, perform () {
e.attr({cx: 513.859 , cy: 35.333, rotation: 0});
run();
});
}
run();
}
tracePath();
Constructing this with Raphaël is a snap. First, you want a base map for the background. Second, you want to create a path to comply with and at last a number of strains of JavaScript to drag all of it collectively.
First we have to create a Raphaël space through which to place all of our SVG. On this instance, we’ll use the myMapHolder div
and create an space that’s 540 pixels broad by 403 pixels tall.
var myMap = raphael("myMapHolder", 540, 403);
Subsequent we’ll drop in a background picture of a map. I used a display shot from Open Avenue Map, however you could possibly use any picture. To verify customers with out JavaScript can nonetheless see the map, we add an <img>
ingredient with a hyperlink to the map picture contained in the myMapHolder div
. The very first thing we do with the JavaScript is take away this baseline picture so the extra dynamic map might be loaded as a replacement.
doc.getElementById("myMapHolder").removeChild(doc.getElementById("myMapHolder").childNodes[0]);
var myImage = myMap.picture("map.png", 0, 0, 540, 403);
I positioned it at zero pixels from the highest and at zero pixels to the left within the holder, then specified the size of the picture itself at 540 by 403 pixels.
var myPath = myMap.path("M513.859,35.222l-95.332,24.667l" +
"18.666,76.667l-131.334,46c0,0-10.667," +
"3.333-11.333,13.333c-0.891,13.371-42.748," +
"23.018-42.748,23.018s-13.252,3.648-9.252," +
"18.315s14.667,43.333,14.667,43.333l-104.667,22.667")
.attr({
stroke: "#f00",
opacity: .7,
"stroke-width": 5
});
Subsequent comes the marginally tough half: The code for the SVG path. I traced the map in Illustrator and saved the trail as an SVG and pasted it in right here, which was the best option to create a posh path rapidly. As it’s also possible to see, we have now connected the attr()
perform to specify the colour, opacity, and width of the trail. This may create a 70% opaque, pink line that’s 5 pixels broad.
var myMarker = myMap.ellipse(513.859,35.333, 7, 7)
.attr({stroke: "none",
opacity: .7,
fill: "#f00"
});
As soon as we’ve arrange the map and the trail, we have to create a small dot to “run the maze.” That is the code that creates a circle with a seven-pixel radius beginning at level 513.859,35.333. We’ve additionally connected some further attributes to take away the border, change the opacity, and the colour of the dot.
perform run() {
myMarker.animateAlong(p, 20000, false, perform () {
myMarker.attr({cx: 513.859 , cy: 35.333, rotation: 0});
run();
});
}
run();
Lastly, we put all of it collectively into an animation. We create a perform referred to as run()
, which strikes the dot alongside the trail. When it’s completed executing, it resets and loops again and again.
Now, to animate the dot, we place it into the variable myMarker and write myMarker.animationAlong()
. This perform takes a number of arguments: First we specify the trail we would like it to comply with, in our case “myPath,” then we specify a length for the size of time we would like the dot to take because it travels the trail. The following argument rotates the article alongside a path. We don’t need this impact, so we set this argument to false. Lastly, we have now a callback perform, which on this case resets the dot to the beginning place to create the loop.
You may see the total script and instance put collectively so you may view supply to adapt it as wanted.
Advantages of SVG on the Internet#section8
As HTML5 grows in recognition, SVG is being rivaled by the <canvas>
ingredient. Each can draw graphics on the display, which makes it complicated to know which to make use of. The most important distinction is that every one the SVG parts are a part of the DOM tree. With canvas
, you may solely entry the foundation <canvas>
ingredient.
Since SVG is a part of the DOM, you may add occasions to all the person parts of an SVG graphic. Actions corresponding to onClick
, onTap
or onHover
all make SVG graphics intractable to the purpose of being a full Flash substitute. Since these occasions might be constructed up at run time, relying on the browser / gadget and its capabilities, it permits for a progressively enhanced expertise. With <canvas>
the drawing shouldn’t be a part of the DOM, and also you sacrifice interactivity for a much less reminiscence intensive DOM tree. Injecting a posh SVG graphic may grind slower machines to a halt as they render hundreds of DOM nodes.
SVG is scalable, in order increasingly browsers transfer to the “zoom” relatively than “scale” mechanism to extend font sizes and graphics, SVG retains its easy edges. The <canvas>
ingredient is rasterized which turns into pixelated.
SVG: a W3C commonplace#section9
SVG is a W3C commonplace, which signifies that nobody goes to take their ball and go residence, leaving you caught with a great deal of ineffective code. Because it’s not solely open, however royalty-free, increasingly purposes, gadgets, and companies are each consuming and merchandising SVG. A viable ecosystem has emerged to the purpose that SVG isn’t going to vanish. It additionally signifies that you don’t want to fret concerning the implementation particulars. The purposes do all of the arduous work, creating the SVG nodes and paths for you. The uncooked XML continues to be there if you wish to poke your head into the code and alter it as wanted.
SVG has been a maturing know-how for a number of years. Now, with the assist of all the main browser producers (together with IE9), it’s at a tipping level. The truth that we’re seeing devoted JavaScript libraries corresponding to Raphaël written by good folks like Dmitry Baranovskiy, is only a signal of what’s to come back. Making progressively enhanced, open-standard, vector-based graphics and animations with easy, scalable curves, and odd-shaped clickable areas makes the way forward for the net fairly thrilling!