Progressive enhancement has turn into a longtime best-practice method to standards-based growth. By beginning with clear, semantic HTML, and layering enhancements utilizing JavaScript and CSS, we try to create a usable expertise for everybody: much less refined units and browsers get an easier however fully useful expertise, whereas extra succesful ones get the bells and whistles.
Article Continues Beneath
That’s the speculation, at the least. However in apply, enhancements are nonetheless delivered to most units, together with those who solely partially perceive them—particularly older browsers and underneath featured cell units. Customers of those units initially obtain a superbly useful web page, nevertheless it’s progressively “enhanced” into a large number of scripts and kinds gone awry, totally defeating the aim of the method.
So how can we construct enhanced experiences whereas ensuring all customers get a useful website? By testing a tool’s capabilities up entrance, we will make knowledgeable choices concerning the stage of expertise to ship to that machine.
Testing for capabilities#section2
Not way back, we discovered that we may check parts of a tool’s CSS assist utilizing easy JavaScript. It began with a easy field mannequin check: we injected a component into the web page, utilized numerous kinds to the ingredient, after which used JavaScript to examine whether or not the ingredient was rendered correctly.
perform boxmodel(){
var newDiv = doc.createElement('div');
doc.physique.appendChild(newDiv);
newDiv.type.width="20px";
newDiv.type.padding = '10px';
var divWidth = newDiv.offsetWidth;
doc.physique.removeChild(newDiv);
return divWidth == 40;
}
Utilizing the perform above, we will pose the query, if(boxmodel())
to search out out if a browser correctly helps the CSS field mannequin. If a characteristic is correctly supported, we will safely improve our web page utilizing this characteristic.
With this concept in thoughts, we wrote extra features to check assist for different CSS properties, reminiscent of:
float
clear
place
overflow
line-height
However testing CSS assist doesn’t cowl the whole lot. Happily, we will additionally use methods reminiscent of object detection to check a browser’s JavaScript assist. We wrote extra features to check generally used JavaScript Objects, reminiscent of:
doc.createElement()
doc.getElementById()
xmlHttpRequest()
window.onresize()
window.print()
We discovered that by operating all the assessments above, we may get a good suggestion of whether or not or not a tool would correctly show the improved model of most of our shoppers’ internet purposes.
Following up on this premise, I, together with my colleagues at Filament Group, developed testUserDevice.js
, which assessments the capabilities of a tool earlier than offering enhancements. This script runs independently of any JavaScript libraries, weighs in at round 5kb, and executes in 5 – 6 milliseconds.
The assessments run by testUserDevice.js
could be modified, added, or deleted to fulfill the necessities of a given web site. There are even choices that assist you to run a number of check instances or a subset of assessments to create multi-level consumer experiences.
Making use of the check outcomes#section3
Integrating testUserDevice.js
right into a web page is easy, simply connect the JavaScript file to your web page and name the next:
testUserDevice.init();
This may inform testUserDevice
to start operating all accessible assessments as quickly because the physique
ingredient is obtainable within the DOM. If all assessments return with a passing rating, the script will proceed to make enhancements.
For the sake of this text, let’s check out a reasonably advanced type with some alternatives for enhancement. First, we begin with probably the most fundamental HTML and a easy linear format.
Demo 1
CSS enhancements#section4
As soon as a tool has efficiently handed a given set of assessments, testUserDevice.js
will allow web page enhancements in various methods. By default, the next DOM modifications are made:
- The category identify
enhanced
is added to the physique ingredient. - Alternate stylesheet hyperlinks with title attributes of (
title=“enhanced”
) are enabled. - Inversely, all stylesheet hyperlinks with title attributes of (
title=“not_enhanced”
) are disabled.
These web page modifications present a number of hooks that may assist you to arrange layers of progressive enhancement. For starters, the physique
class can be utilized together with CSS selectors to make small expertise enhancements. For instance, you might need three div
s which might be stacked vertically at web page load:
physique div.instance {
margin: 1em 0;
}
If the consumer’s browser or machine passes the check, these div
s might be repositioned into three floated columns:
physique.enhanced div.instance {
float: left;
width: 30%;
margin: 0;
}
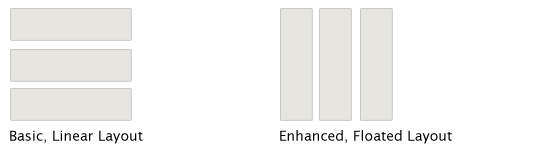
This works nicely for pages that comprise just a few enhancements, nevertheless it wouldn’t make sense to write down a whole superior stylesheet utilizing these conditional selectors. For giant enhancements, you possibly can retailer superior kinds in alternate stylesheets. By giving these alternate stylesheets a title of enhanced
, they are going to be enabled within the occasion of a handed check. For instance, this:
<hyperlink rel="alternate stylesheet" kind="textual content/css" href="https://alistapart.com/article/testdriven/enhanced.css" title="enhanced" />
turns into this:
<hyperlink rel="stylesheet" kind="textual content/css" href="https://alistapart.com/article/testdriven/enhanced.css" title="enhanced" />
In fact, that is nothing greater than a fundamental stylesheet change, however the necessary level is that we’ve arrange our enhancements to indicate solely in units that may deal with them correctly.
As talked about earlier, testUserDevice.js
will even disable any stylesheets specified by a title attribute of (title="not_enhanced"
). This is probably not essential typically, however you probably have fundamental stylesheets that may battle with the improved ones, this can be a good approach to hold them separated.
The next demonstration revisits our type instance, now with a format that’s progressively enhanced with CSS in succesful units.
Demo 2
Though the demo above makes use of comparatively easy CSS, it’s nonetheless necessary to check for machine capabilities first for the reason that format could also be unusable in a tool that renders the CSS improperly.
JavaScript enhancements#section5
Many web page enhancements use JavaScript. Happily, testUserDevice.js
lets you specify scripting that you simply wish to execute in your enhanced expertise as nicely. That is executed by merely passing in a perform as an argument into our script, as follows.
testUserDevice.init( perform(){ /* fancy stuff goes right here */ } );
As you possibly can see, we’ve handed an nameless perform as an argument to our init
methodology. If our check passes, the scripting enclosed within the perform argument will execute instantly. Remember that that is prone to happen earlier than your typical JavaScript library’s DOM prepared occasion will fireplace, so DOM-related scripting ought to nonetheless be enclosed in an occasion that waits for parts to be accessible earlier than executing (reminiscent of jQuery’s prepared
occasion or the physique.onload
occasion).
Our ultimate demo web page shows the identical type as earlier than, now progressively enhanced utilizing JavaScript (in units deemed succesful) to its full characteristic set:
- The birthday subject has a date-picker part.
- The choice choices at the moment are sliders.
- Within the favourite pet
fieldset
, the “breed” fields are conditionally enabled by the chosen radio merchandise. - The “breed” fields use auto-complete.
- The shape now makes use of Ajax for submission.
Demo 3
As soon as is all you want…#section6
Upon the completion of a check, testUserDevice.js
drops a cookie recording the outcomes of every check case and makes use of it on future web page masses, which suggests much less processing and higher efficiency for customers. However the alternatives to optimize don’t cease on the entrance finish: with just a little PHP useful work, you possibly can really search for these cookies on the back-end and serve pages understanding a tool’s capabilities already! This implies you would serve your web page with an enhanced
class already in your physique ingredient, allow your superior stylesheets from the beginning, and execute superior scripting instantly at web page load.
<?php //examine for cookie (enhanced=move), and set the stylesheet rel relying on its worth
$cssRel = ($_COOKIE['enhanced'] == "move") ? "stylesheet" : "alternate stylesheet"; echo '<hyperlink rel="' . $cssRel . '" kind="textual content/css" href="https://alistapart.com/article/testdriven/css/enhanced.css" title="enhanced" />'; ?>
testUserDevice.js
contains choices for dealing with customized check instances and including your individual assessments.
Writing customized check instances#section8
Utilizing testUserDevice
’s default habits of operating a single check case is prone to be sufficient for many initiatives, however in case you’d prefer to make a number of expertise divisions, the choice is obtainable. For instance, if we supposed to make solely two enhancements on our demo web page:
- Kind submission through ajax
- Choose menus are transformed into sliders
By default, we’d check for all the options required for these enhancements, after which improve your entire web page if the machine passes all of the assessments. In distinction, we might want Ajax to work no matter whether or not the selects are sliders or not (and vice versa). That is what I name a number of expertise divisions. For this, we’ll want to write down a customized check case, reminiscent of the next case which solely assessments a tool’s Ajax assist.
testUserDevice.init([
{
testName: 'ajaxCapable',
pass: ['ajax'],
scripting: perform(){ useAjax(); }
}
]);
As a substitute of passing a perform into our init
perform as we did earlier, we’re now passing an array as an argument as a substitute. This array incorporates a number of objects every containing three settings: testName
(string, identify of check), move
(array of check names comparable to accessible assessments), and scripting
(perform to execute upon passing check case). These check case objects can be utilized to make enhancements that solely require a subset of options, letting you skip the all-or-nothing check.
Remember that every check case’s testName property might be used to make DOM modifications in the identical approach our default check used the identify “enhanced”. Which means upon passing check case above, our script will add a category of ajaxCapable
to the physique
ingredient, stylesheets might be enabled and disabled primarily based on title attributes containing the phrase ajaxCapable
, and a cookie might be saved as ajaxCapable=move
.
Including your individual assessments#section9
The entire assessments included in testUserDevice.js
are saved in a assessments
object that may be simply modified to your wants. testUserDevice.js
additionally comes with a useful helper perform to allow you to add assessments in a clear, separated method. This helper perform could be reached by calling testUserDevice.add(testName, testScript)
. For instance, so as to add a check that makes positive a consumer’s browser helps a easy JavaScript affirmation, you would write:
testUserDevice.add( 'affirm', perform(){
if(window.affirm){return true;}
else {return false;}
});
Or, extra concisely…
testUserDevice.add( 'affirm', perform(){
return (window.affirm) ? true : false;
});
The add
perform’s first argument incorporates a string representing the identify of this check. The second argument is the check itself, which should be a perform that returns true or false (true for a passing grade; false for failure).
Growing for tomorrow, as we speak#section10
Because the machine and browser panorama continues to develop and builders proceed to search out methods to implement extra options and functionalities, our job of creating universally usable web sites will stay difficult. Integrating capabilities testing into our growth course of permits us to take full benefit of state-of-the-art options with out ruining the expertise for the customers of much less succesful browsers and units.
It’s necessary to do not forget that the method described on this article doesn’t endorse using any explicit set of assessments—simply the concept that you need to check to ensure capabilities exist earlier than making an attempt to make use of them. We welcome any concepts and recommendations on how our method could be improved and prolonged.