We’ve all been there: that little bit of JavaScript performance that started off as only a handful of traces grows to a dozen, then two dozen, then extra. Alongside the way in which, a perform picks up a couple of extra arguments; a conditional picks up a couple of extra situations. After which someday, the bug report is available in: one thing’s damaged, and it’s as much as us to untangle the mess.
Article Continues Under
As we ask our client-side code to tackle an increasing number of duties—certainly, entire functions live largely within the browser as of late—two issues have gotten clear. One, we will’t simply level and click on our method via testing that issues are working as we anticipate; automated checks are key to having confidence in our code. Two, we’re in all probability going to have to vary how we write our code to be able to make it attainable to jot down checks.
Actually, we have to change how we code? Sure—as a result of even when we all know that automated checks are a superb factor, most of us are in all probability solely in a position to write integration checks proper now. Integration checks are worthwhile as a result of they give attention to how the items of an utility work collectively, however what they don’t do is inform us whether or not particular person models of performance are behaving as anticipated.
That’s the place unit testing is available in. And we’ll have a really laborious time writing unit checks till we begin writing testable JavaScript.
Unit vs. integration: what’s the distinction?#section2
Writing integration checks is normally pretty easy: we merely write code that describes how a consumer interacts with our app, and what the consumer ought to anticipate to see as she does. Selenium is a well-liked device for automating browsers. Capybara for Ruby makes it straightforward to speak to Selenium, and there are many instruments for different languages, too.
Right here’s an integration check for a portion of a search app:
def test_search
fill_in('q', :with => 'cat')
discover('.btn').click on
assert( discover('#outcomes li').has_content?('cat'), 'Search outcomes are proven' )
assert( web page.has_no_selector?('#outcomes li.no-results'), 'No outcomes shouldn't be proven' )
finish
Whereas an integration check is all for a consumer’s interplay with an app, a unit check is narrowly targeted on a small piece of code:
After I name a perform with a sure enter, do I obtain the anticipated output?
Apps which might be written in a conventional procedural fashion will be very tough to unit check—and tough to take care of, debug, and lengthen, too. But when we write our code with our future unit testing wants in thoughts, we is not going to solely discover that writing the checks turns into extra easy than we’d have anticipated, but additionally that we’ll merely write higher code, too.
To see what I’m speaking about, let’s check out a easy search app:
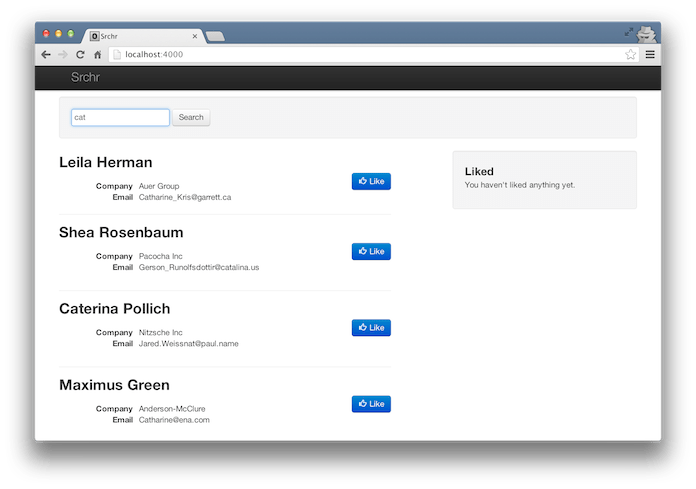
When a consumer enters a search time period, the app sends an XHR to the server for the corresponding information. When the server responds with the info, formatted as JSON, the app takes that information and shows it on the web page, utilizing client-side templating. A consumer can click on on a search consequence to point that he “likes” it; when this occurs, the title of the individual he preferred is added to the “Favored” record on the right-hand aspect.
A “conventional” JavaScript implementation of this app may seem like this:
var tmplCache = {};
perform loadTemplate (title) {
if (!tmplCache[name]) {
tmplCache[name] = $.get('/templates/' + title);
}
return tmplCache[name];
}
$(perform () {
var resultsList = $('#outcomes');
var preferred = $('#preferred');
var pending = false;
$('#searchForm').on('submit', perform (e) {
e.preventDefault();
if (pending) { return; }
var type = $(this);
var question = $.trim( type.discover('enter[name="q"]').val() );
if (!question) { return; }
pending = true;
$.ajax('/information/search.json', {
information : { q: question },
dataType : 'json',
success : perform (information) {
loadTemplate('people-detailed.tmpl').then(perform (t) {
var tmpl = _.template(t);
resultsList.html( tmpl({ individuals : information.outcomes }) );
pending = false;
});
}
});
$('<li>', {
'class' : 'pending',
html : 'Looking …'
}).appendTo( resultsList.empty() );
});
resultsList.on('click on', '.like', perform (e) {
e.preventDefault();
var title = $(this).closest('li').discover('h2').textual content();
preferred.discover('.no-results').take away();
$('<li>', { textual content: title }).appendTo(preferred);
});
});
My buddy Adam Sontag calls this Select Your Personal Journey code—on any given line, we is perhaps coping with presentation, or information, or consumer interplay, or utility state. Who is aware of! It’s straightforward sufficient to jot down integration checks for this sort of code, however it’s laborious to check particular person models of performance.
What makes it laborious? 4 issues:
- A common lack of construction; nearly all the things occurs in a
$(doc).prepared()
callback, after which in nameless capabilities that may’t be examined as a result of they aren’t uncovered. - Complicated capabilities; if a perform is greater than 10 traces, just like the submit handler, it’s extremely probably that it’s doing an excessive amount of.
- Hidden or shared state; for instance, since
pending
is in a closure, there’s no technique to check whether or not the pending state is about accurately. - Tight coupling; for instance, a
$.ajax
success handler shouldn’t want direct entry to the DOM.
Organizing our code#section3
Step one towards fixing that is to take a much less tangled method to our code, breaking it up into a couple of totally different areas of accountability:
- Presentation and interplay
- Information administration and persistence
- General utility state
- Setup and glue code to make the items work collectively
Within the “conventional” implementation proven above, these 4 classes are intermingled—on one line we’re coping with presentation, and two traces later we is perhaps speaking with the server.
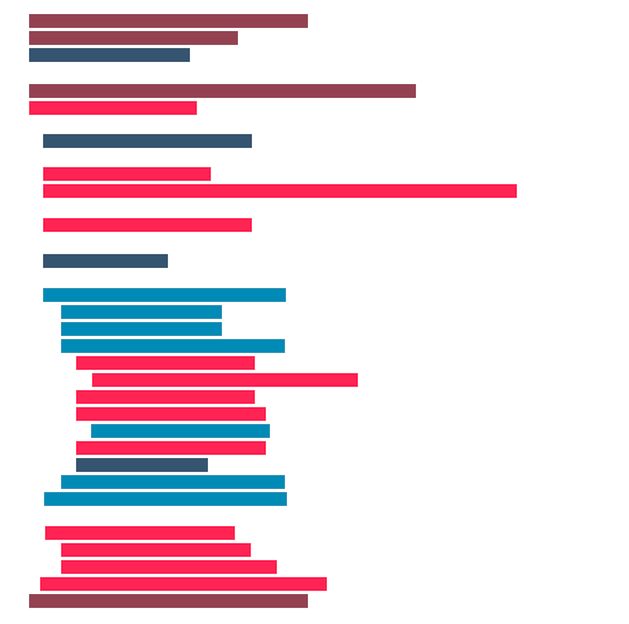
Whereas we will completely write integration checks for this code—and we must always!—writing unit checks for it’s fairly tough. In our practical checks, we will make assertions akin to “when a consumer searches for one thing, she ought to see the suitable outcomes,” however we will’t get rather more particular. If one thing goes incorrect, we’ll have to trace down precisely the place it went incorrect, and our practical checks gained’t assist a lot with that.
If we rethink how we write our code, although, we will write unit checks that may give us higher perception into the place issues went incorrect, and likewise assist us find yourself with code that’s simpler to reuse, keep, and lengthen.
Our new code will comply with a couple of guiding ideas:
- Signify every distinct piece of conduct as a separate object that falls into one of many 4 areas of accountability and doesn’t must find out about different objects. This may assist us keep away from creating tangled code.
- Help configurability, reasonably than hard-coding issues. This may stop us from replicating our whole HTML atmosphere to be able to write our checks.
- Hold our objects’ strategies easy and transient. This may assist us maintain our checks easy and our code straightforward to learn.
- Use constructor capabilities to create situations of objects. This may make it attainable to create “clear” copies of every piece of code for the sake of testing.
To start out with, we have to work out how we’ll break our utility into totally different items. We’ll have three items devoted to presentation and interplay: the Search Type, the Search Outcomes, and the Likes Field.
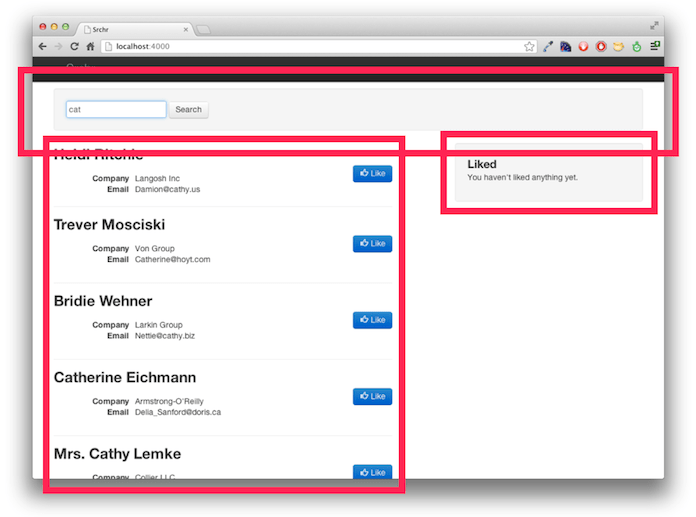
We’ll even have a chunk devoted to fetching information from the server and a chunk devoted to gluing all the things collectively.
Let’s begin by taking a look at one of many easiest items of our utility: the Likes Field. Within the unique model of the app, this code was chargeable for updating the Likes Field:
var preferred = $('#preferred');
var resultsList = $('#outcomes');
// ...
resultsList.on('click on', '.like', perform (e) {
e.preventDefault();
var title = $(this).closest('li').discover('h2').textual content();
preferred.discover( '.no-results' ).take away();
$('<li>', { textual content: title }).appendTo(preferred);
});
The Search Outcomes piece is totally intertwined with the Likes Field piece and must know quite a bit about its markup. A significantly better and extra testable method could be to create a Likes Field object that’s chargeable for manipulating the DOM associated to the Likes Field:
var Likes = perform (el) {
this.el = $(el);
return this;
};
Likes.prototype.add = perform (title) {
this.el.discover('.no-results').take away();
$('<li>', { textual content: title }).appendTo(this.el);
};
This code offers a constructor perform that creates a brand new occasion of a Likes Field. The occasion that’s created has an .add()
technique, which we will use so as to add new outcomes. We will write a few checks to show that it really works:
var ul;
setup(perform(){
ul = $('<ul><li class="no-results"></li></ul>');
});
check('constructor', perform () {
var l = new Likes(ul);
assert(l);
});
check('including a reputation', perform () {
var l = new Likes(ul);
l.add('Brendan Eich');
assert.equal(ul.discover('li').size, 1);
assert.equal(ul.discover('li').first().html(), 'Brendan Eich');
assert.equal(ul.discover('li.no-results').size, 0);
});
Not so laborious, is it? Right here we’re utilizing Mocha because the check framework, and Chai because the assertion library. Mocha offers the check
and setup
capabilities; Chai offers assert
. There are many different check frameworks and assertion libraries to select from, however for the sake of an introduction, I discover these two work nicely. You must discover the one which works finest for you and your venture—apart from Mocha, QUnit is widespread, and Intern is a brand new framework that reveals quite a lot of promise.
Our check code begins out by creating a component that we’ll use because the container for our Likes Field. Then, it runs two checks: one is a sanity verify to verify we will make a Likes Field; the opposite is a check to make sure that our .add()
technique has the specified impact. With these checks in place, we will safely refactor the code for our Likes Field, and be assured that we’ll know if we break something.
Our new utility code can now seem like this:
var preferred = new Likes('#preferred');
var resultsList = $('#outcomes');
// ...
resultsList.on('click on', '.like', perform (e) {
e.preventDefault();
var title = $(this).closest('li').discover('h2').textual content();
preferred.add(title);
});
The Search Outcomes piece is extra complicated than the Likes Field, however let’s take a stab at refactoring that, too. Simply as we created an .add()
technique on the Likes Field, we additionally need to create strategies for interacting with the Search Outcomes. We’ll need a method so as to add new outcomes, in addition to a technique to “broadcast” to the remainder of the app when issues occur inside the Search Outcomes—for instance, when somebody likes a consequence.
var SearchResults = perform (el) {
this.el = $(el);
this.el.on( 'click on', '.btn.like', _.bind(this._handleClick, this) );
};
SearchResults.prototype.setResults = perform (outcomes) {
var templateRequest = $.get('people-detailed.tmpl');
templateRequest.then( _.bind(this._populate, this, outcomes) );
};
SearchResults.prototype._handleClick = perform (evt) {
var title = $(evt.goal).closest('li.consequence').attr('data-name');
$(doc).set off('like', [ name ]);
};
SearchResults.prototype._populate = perform (outcomes, tmpl) {
var html = _.template(tmpl, { individuals: outcomes });
this.el.html(html);
};
Now, our outdated app code for managing the interplay between Search Outcomes and the Likes Field may seem like this:
var preferred = new Likes('#preferred');
var resultsList = new SearchResults('#outcomes');
// ...
$(doc).on('like', perform (evt, title) {
preferred.add(title);
})
It’s a lot easier and fewer entangled, as a result of we’re utilizing the doc
as a worldwide message bus, and passing messages via it so particular person parts don’t must find out about one another. (Notice that in an actual app, we’d use one thing like Spine or the RSVP library to handle occasions. We’re simply triggering on doc
to maintain issues easy right here.) We’re additionally hiding all of the soiled work—akin to discovering the title of the one who was preferred—contained in the Search Outcomes object, reasonably than having it muddy up our utility code. The perfect half: we will now write checks to show that our Search Outcomes object works as we anticipate:
var ul;
var information = [ /* fake data here */ ];
setup(perform () {
ul = $('<ul><li class="no-results"></li></ul>');
});
check('constructor', perform () {
var sr = new SearchResults(ul);
assert(sr);
});
check('show obtained outcomes', perform () {
var sr = new SearchResults(ul);
sr.setResults(information);
assert.equal(ul.discover('.no-results').size, 0);
assert.equal(ul.discover('li.consequence').size, information.size);
assert.equal(
ul.discover('li.consequence').first().attr('data-name'),
information[0].title
);
});
check('announce likes', perform() {
var sr = new SearchResults(ul);
var flag;
var spy = perform () {
flag = [].slice.name(arguments);
};
sr.setResults(information);
$(doc).on('like', spy);
ul.discover('li').first().discover('.like.btn').click on();
assert(flag, 'occasion handler known as');
assert.equal(flag[1], information[0].title, 'occasion handler receives information' );
});
The interplay with the server is one other attention-grabbing piece to think about. The unique code included a direct $.ajax()
request, and the callback interacted straight with the DOM:
$.ajax('/information/search.json', {
information : { q: question },
dataType : 'json',
success : perform( information ) {
loadTemplate('people-detailed.tmpl').then(perform(t) {
var tmpl = _.template( t );
resultsList.html( tmpl({ individuals : information.outcomes }) );
pending = false;
});
}
});
Once more, that is tough to jot down a unit check for, as a result of so many various issues are occurring in only a few traces of code. We will restructure the info portion of our utility as an object of its personal:
var SearchData = perform () { };
SearchData.prototype.fetch = perform (question) {
var dfd;
if (!question) {
dfd = $.Deferred();
dfd.resolve([]);
return dfd.promise();
}
return $.ajax( '/information/search.json', {
information : { q: question },
dataType : 'json'
}).pipe(perform( resp ) {
return resp.outcomes;
});
};
Now, we will change our code for getting the outcomes onto the web page:
var resultsList = new SearchResults('#outcomes');
var searchData = new SearchData();
// ...
searchData.fetch(question).then(resultsList.setResults);
Once more, we’ve dramatically simplified our utility code, and remoted the complexity inside the Search Information object, reasonably than having it reside in our essential utility code. We’ve additionally made our search interface testable, although there are a pair caveats to remember when testing code that interacts with the server.
The primary is that we don’t need to truly work together with the server—to take action could be to reenter the world of integration checks, and since we’re accountable builders, we have already got checks that make sure the server does the best factor, proper? As an alternative, we need to “mock” the interplay with the server, which we will do utilizing the Sinon library. The second caveat is that we also needs to check non-ideal paths, akin to an empty question.
check('constructor', perform () {
var sd = new SearchData();
assert(sd);
});
suite('fetch', perform () {
var xhr, requests;
setup(perform () {
requests = [];
xhr = sinon.useFakeXMLHttpRequest();
xhr.onCreate = perform (req) {
requests.push(req);
};
});
teardown(perform () {
xhr.restore();
});
check('fetches from appropriate URL', perform () {
var sd = new SearchData();
sd.fetch('cat');
assert.equal(requests[0].url, '/information/search.json?q=cat');
});
check('returns a promise', perform () {
var sd = new SearchData();
var req = sd.fetch('cat');
assert.isFunction(req.then);
});
check('no request if no question', perform () {
var sd = new SearchData();
var req = sd.fetch();
assert.equal(requests.size, 0);
});
check('return a promise even when no question', perform () {
var sd = new SearchData();
var req = sd.fetch();
assert.isFunction( req.then );
});
check('no question promise resolves with empty array', perform () {
var sd = new SearchData();
var req = sd.fetch();
var spy = sinon.spy();
req.then(spy);
assert.deepEqual(spy.args[0][0], []);
});
check('returns contents of outcomes property of the response', perform () {
var sd = new SearchData();
var req = sd.fetch('cat');
var spy = sinon.spy();
requests[0].reply(
200, { 'Content material-type': 'textual content/json' },
JSON.stringify({ outcomes: [ 1, 2, 3 ] })
);
req.then(spy);
assert.deepEqual(spy.args[0][0], [ 1, 2, 3 ]);
});
});
For the sake of brevity, I’ve omitted the refactoring of the Search Type, and likewise simplified among the different refactorings and checks, however you’ll be able to see a completed model of the app right here in the event you’re .
After we’re performed rewriting our utility utilizing testable JavaScript patterns, we find yourself with one thing a lot cleaner than what we began with:
$(perform() {
var pending = false;
var searchForm = new SearchForm('#searchForm');
var searchResults = new SearchResults('#outcomes');
var likes = new Likes('#preferred');
var searchData = new SearchData();
$(doc).on('search', perform (occasion, question) {
if (pending) { return; }
pending = true;
searchData.fetch(question).then(perform (outcomes) {
searchResults.setResults(outcomes);
pending = false;
});
searchResults.pending();
});
$(doc).on('like', perform (evt, title) {
likes.add(title);
});
});
Much more necessary than our a lot cleaner utility code, although, is the truth that we find yourself with a codebase that’s completely examined. Which means we will safely refactor it and add to it with out the worry of breaking issues. We will even write new checks as we discover new points, after which write the code that makes these checks cross.
Testing makes life simpler in the long term#section4
It’s straightforward to take a look at all of this and say, “Wait, you need me to jot down extra code to do the identical job?”
The factor is, there are a couple of inescapable information of life about Making Issues On The Web. You’ll spend time designing an method to an issue. You’ll check your answer, whether or not by clicking round in a browser, writing automated checks, or—shudder—letting your customers do your testing for you in manufacturing. You’ll make adjustments to your code, and different individuals will use your code. Lastly: there will probably be bugs, regardless of what number of checks you write.
The factor about testing is that whereas it would require a bit extra time on the outset, it actually does save time in the long term. You’ll be patting your self on the again the primary time a check you wrote catches a bug earlier than it finds its method into manufacturing. You’ll be grateful, too, when you will have a system in place that may show that your bug repair actually does repair a bug that slips via.
Further sources#section5
This text simply scratches the floor of JavaScript testing, however in the event you’d prefer to be taught extra, take a look at:
- My presentation from the 2012 Full Frontal convention in Brighton, UK.
- Grunt, a device that helps automate the testing course of and many different issues.
- Take a look at-Pushed JavaScript Growth by Christian Johansen, the creator of the Sinon library. It’s a dense however informative examination of the observe of testing JavaScript.