When used successfully, JavaScript debuggers assist discover and squash errors in your JavaScript code. To turn out to be a sophisticated JavaScript debugger, you’ll have to know in regards to the debuggers out there to you, the everyday JavaScript debugging workflow, and code necessities for efficient debugging. On this article, we’ll focus on superior debugging strategies for diagnosing and treating bugs utilizing a pattern net software.
Article Continues Beneath
On accessibility#section2
This text highlights strengths and variations between debugging instruments, and reveals how we carry out superior JavaScript debugging duties. Our strategies typically depend on the mouse; should you want to make use of keyboard shortcuts, or should you depend on assistive applied sciences corresponding to display screen readers to work together with these instruments, it is best to seek the advice of the related documentation to find out how (or if) these instruments will give you the results you want.
With an growing choice of good debuggers out there, JavaScript programmers can achieve rather a lot from studying how you can use them. JavaScript debugger consumer interfaces (UIs) have gotten extra polished, extra standardized throughout merchandise, and simpler to make use of, thus making it simpler for each consultants and novices to be taught JavaScript debugging.
At the moment, there are debugging instruments out there for all main net browsers.
At the moment, Firebug and Dragonfly are essentially the most secure choices. IE8’s beta instruments generally ignore breakpoints, and on the time this text was written, WebInspector appeared to fit points with the nightly builds of Webkit.
Familiarize your self with a number of debugging instruments—you by no means know wherein browser the subsequent bug will come up. For the reason that debuggers are roughly comparable in performance, it’s simple to modify between them as soon as you understand how to make use of one.
When investigating a particular drawback, you’ll normally observe this course of:
- Discover the related code within the debugger’s code view pane.
- Set breakpoint(s) the place you suppose fascinating issues could happen.
- Run the script once more by reloading the web page within the browser if it’s an inline script, or by clicking a button if it’s an occasion handler.
- Wait till the debugger pauses execution and makes it attainable to step via the code.
- Examine the values of variables. For instance, search for variables which are undefined when they need to comprise a price, or return “false” once you anticipate them to return “true.”
- If essential, use the command line to judge code or change variables for testing.
- Discover the issue by studying which piece of code or enter induced the error situations.
To create a breakpoint, you too can add the assertion debugger
to your code:
operate frmSubmit(occasion)
Debugger necessities#section5
Most debuggers require well-formatted code. Scripts written on one line make it tough to identify errors in line-by-line debuggers. Obfuscated code will be onerous to debug, particularly code that has been “packed” and must be unpacked utilizing eval()
. Many JavaScript libraries help you select between packed/obfuscated and well-formatted variations, making it simple to make use of formatted code for debugging.
Let’s begin with a small, bug-ridden instance, to discover ways to diagnose and deal with every ailment in flip. Our instance is an internet software login display screen.
Think about you’re engaged on this fabulous new net software, and your testers ask you to analyze this record of bugs:
- The “Loading…” standing bar message doesn’t disappear when the appliance finishes loading.
- The language defaults to Norwegian even in English variations of Firefox and IE.
- A worldwide variable named
prop
is created someplace. - Within the DOM viewer, all parts have a “
clone
” attribute. - “Minimal size” kind validation doesn’t work—making an attempt to submit the shape with a one-letter consumer identify ought to trigger an error message.
- Leaving the password subject clean is allowed—it is best to see an error message saying that the password subject is probably not empty, on submitting the shape.
- The login all the time fails, with an error message saying {that a} cross-site request forgery assault was detected.
Launching debuggers#section7
- In Firefox you’ll want to be sure to have the Firebug extension put in. Choose “Instruments > Firebug > Open Firebug” to get began.
- In Opera 9.5 beta 2 or later, select “Instruments > Superior > Developer Instruments.”
- In IE8 beta, select “Instruments > Developer Instruments.”
- In Safari or WebKit, first allow the debug thực đơn, then select “Debug > Present Internet Inspector.”
It’s time to fireside up the debuggers. To observe the demo, pay shut consideration to the step-by-step directions all through the rest of the article. Since some directions contain modifying the code, you might wish to save the web page regionally and cargo it out of your file system earlier than beginning.
Bug one: the “Loading…” message within the standing bar#section8
For those who take a look at the debugging purposes in Dragonfly and Firebug, you’ll get an preliminary view as seen in determine one.
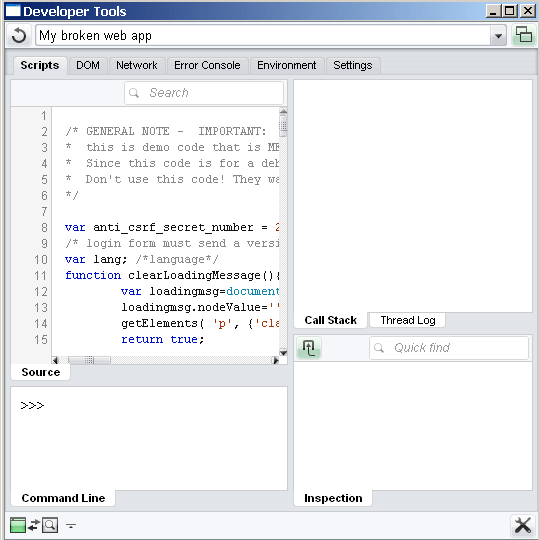
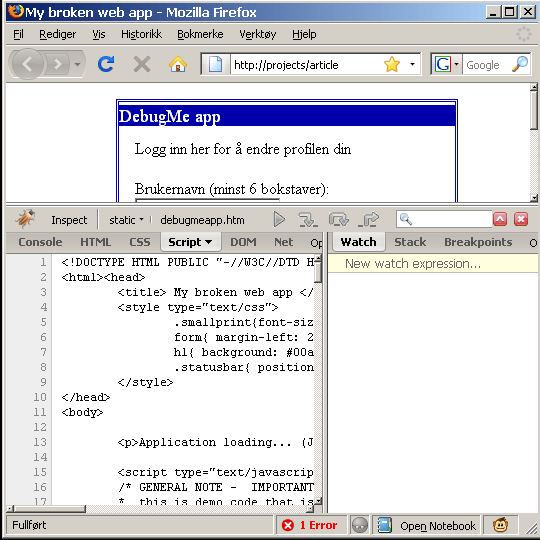
fig. 1: the preliminary view of our software’s JavaScript in Dragonfly and Firebug, respectively.
Once you take a look at the supply code within the debugger, observe that there’s a operate clearLoadingMessage()
outlined on the high of the code. This looks as if a great place to set a breakpoint.
Right here’s how you can do it:
- Click on the road quantity within the left margin to set a breakpoint on the primary line contained in the operate
clearLoadingMessage()
. - Reload the web page.
Notice: the breakpoint should be set on a line with code that can execute when the operate runs. The road that incorporates operate clearLoadingMessage(){
is simply the operate signature. Setting a breakpoint there’ll by no means really trigger the debugger to cease. Set your breakpoint on the primary line contained in the operate as an alternative.
When the web page is reloaded, script execution stops on the breakpoint and also you’ll see an output just like the one proven in determine two. (Dragonfly is proven on the high, Firebug on the backside.)


fig. 2: the debuggers stopped at a breakpoint inside clearLoadingMessage
.
Let’s step via the operate. You’ll see that it updates two DOM parts, and on line 28 it mentions the phrase statusbar
. That appears important. It’s seemingly that getElements( ‘p’, {'class':'statusbar'} )[0]
will discover the statusbar
aspect within the DOM. Is there a method you may take a look at that idea shortly?
Paste the related snippet into the command line to examine your idea. Determine three reveals three screenshots (Dragonfly, Firebug, and IE8) after studying the innerHTML
or outerHTML
of the aspect returned by the command you’re investigating.
To check this assumption:
- Discover the command line:
- In Firebug, swap to the “Console” tab.
- In Dragonfly, look under the JavaScript supply code pane.
- In IE8 Developer Instruments, discover the tab on the fitting labeled “Console.”
- Paste
getElements( ‘p’, {'class':'statusbar'} )[0].innerHTML
into the command line. - Press enter.



fig. 3: output proven in Dragonfly, Firebug, and IE8, respectively.
The command line is a really useful gizmo that permits you to take a look at small script snippets shortly. Firebug’s console integration may be very helpful—in case your command outputs an object, you get an clever view. For instance, you get a markup-like illustration if it’s a DOM object.
You should utilize the command line to discover the issue in additional depth. The road of JavaScript we’re does the next three issues:
- It will get a reference to the standing bar aspect. Within the DOM inspector view, you will note that the corresponding markup is
<p class=“statusbar”>
. - It seems up its
firstChild
, in different phrases, the primary node inside this paragraph aspect. - It units the
innerText
property.
Let’s attempt to run a bit extra of that command from the command line. (Tip: use the up arrow key to navigate again to earlier traces typed into the command line subject.) For instance, you may surprise what the present worth of this aspect’s innerText
property is earlier than it’s set. To examine this, you may kind all the command as much as the equals signal into the command line:
getElements( ‘p’, {'class':'statusbar'} )[0].firstChild.innerText
Surprisingly, the output is…nothing. So the expression getElements( ‘p’, {'class':'statusbar'} )[0].firstChild
factors to one thing within the DOM that doesn’t comprise any textual content, or doesn’t have an innerText
property.
So, the subsequent query is: what precisely is the primary youngster node of the paragraph aspect? Let’s ask the command line that query. (See determine 4 for the outcome.)
![Dragonfly debugger command line outputs [object Text]](https://techacode.com/wp-content/uploads/2023/05/df1-4000.png)
fig. 4: the Dragonfly debugger command line, outputting [object Text]
.
Dragonfly’s [object Text]
output reveals that this can be a DOM textual content node. Firebug reveals us the content material of the textual content node as a hyperlink to the DOM explorer. You will have now discovered the issue that causes bug primary: a textual content node doesn’t have an innerText
property—solely aspect nodes do. Therefore, setting p.firstChild.innerText
does nothing. This bug will be fastened by changing innerText
with nodeValue
, which is a property the W3C DOM normal defines on textual content nodes.
After you’ve had an opportunity to evaluation this instance:
- Press [F5] or the run button to complete the script, now that you simply’ve discovered the issue.
- Keep in mind to clear the previous breakpoint by clicking the road quantity once more.
Bug two: problematic language detection#section9
You’ll have seen the var lang; /*language*/
line close to the highest of the JavaScript—the code that units this variable might be concerned when issues go improper. You’ll find issues within the code shortly utilizing the helpful search capabilities supplied by each debuggers. In Dragonfly it’s simply above the code viewer; in Firebug it’s on the highest proper aspect of the Firebug consumer interface. (See determine 5.)
To seek out the place that offers with the appliance’s localization:
- Sort
lang =
into the search subject. - Set a breakpoint on the road the place the
lang
variable is assigned a price. - Reload the web page.
Safari’s WebInspector additionally has a really highly effective search function. WebInspector permits you to search all of the code on the identical time, together with markup, CSS, and JavaScript. The outcomes are proven in a devoted pane the place you may doubleclick them to leap to the supply line, as proven within the screenshot.

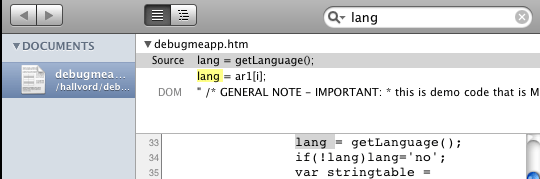
fig. 5: looking with the Dragonfly and WebInspector debuggers.
To examine what this operate does:
- Use the “step into” button to enter the
getLanguage
operate. - Click on the “step into” button repeatedly to undergo the code one line at a time.
- Keep watch over the overview of native variables to see how they modify when you step via the operate.
Moving into the getLanguage
operate, you will note that it tries studying the language from the user-agent string. The writer of this code seen that some language info is included within the user-agent string on some browsers, and tries to parse navigator.userAgent
to extract this info:
var str1 = navigator.userAgent.match( /((.*))/ )[1];
var ar1 = str1.break up(/s*;s*/), lang;
for (var i = 0; i < ar1.size; i++){
if (ar1<i>.match(/^(.{2})$/)){
lang = ar1<i>;
}
}
Whereas stepping via this code with the debuggers, you should use the native variables overview. Determine six reveals Firebug and IE8 Developer Instruments with the ar1
array expanded to indicate the weather in it:
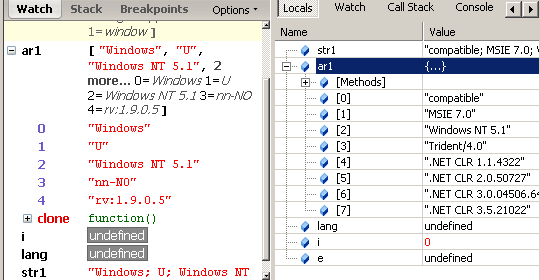
fig. 6: Firebug and IE8’s native variable panes, whereas operating the getLanguage
operate.
The ar1<i>.match(/^(.{2})$/)
statements merely search for a string that’s precisely two characters lengthy, anticipating a two-character language code corresponding to en or no. Nonetheless, as you may see from the screenshots, Firefox’s language info within the user-agent string is definitely nn-NO. IE has no language-related info on this a part of the userAgent
string.
The second bug has been discovered: the language detection seems for a two-letter language code, however Firefox has a 5 letter locale string and IE has nothing. This “language detection” code ought to most likely be scrapped altogether, and changed with one thing server-side that makes use of the HTTP Settle for-Language
header or probably falls again to studying navigator.language
. Or navigator.userLanguage
in IE. One instance of what that operate may appear like is proven under:
operate getLanguage() {
var lang; if (navigator.language) {
lang = navigator.language;
} else if (navigator.userLanguage) {
lang = navigator.userLanguage;
} if (lang && lang.size > 2) {
lang = lang.substring(0, 2);
} return lang;
}
Bug three: the thriller prop
variable#section10
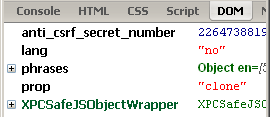
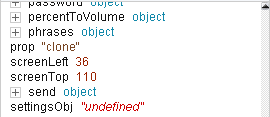
fig. 7: Firebug and Dragonfly’s native variable panes displaying the worldwide prop
variable.
In determine seven, you may clearly see the thriller prop
variable. Effectively-written purposes maintain the variety of world variables to a minimal, as a result of they’ll trigger confusion when totally different sections of the appliance attempt to use the identical variable identify. Think about that tomorrow, one other workforce of builders added a brand new function to our software and likewise named a variable prop
. We’d have two totally different elements of our software making an attempt to make use of the identical variable identify for various issues. This follow is a recipe for conflicts and bugs down the street. Therefore, it’s important to monitor down the place this variable is about and see if there’s a solution to make it an area variable. You may begin looking like we did to seek out bug two above, however maybe there’s a smarter method…
Debuggers for a lot of different programming languages have a “watch” idea that may break into the debugger when a variable adjustments. Neither Dragonfly nor Firebug at the moment assist “watch” but it surely’s simple to get the identical impact by including the next line of debugging code on the high of the supply of the script you’re troubleshooting:
__defineSetter__(‘prop’, operate() { debugger; });
So as to add this “watch”-like performance to the debugged script:
- Add the debugging code to the highest of the primary script.
- Reload the web page.
- Notice the way it breaks when the issue happens.
Utilizing getters and setters can emulate “watch” performance and make it easier to set “sensible” breakpoints.
The IE8 Developer Instruments have a “watch” pane, but it surely doesn’t break when a watched variable is modified. Given IE8’s incomplete assist for getters and setters, you may’t emulate this performance the best way you may in Firefox, Opera, and Safari.
Once you reload the appliance, it’s going to instantly break the place the worldwide variable prop
is being outlined. It really stops on the line of debugging code you added as a result of that is the place the debugger
assertion is. One click on on the “step out” button will take you from the setter operate to the place the place the variable is about. This code is discovered contained in the getElements
operate: (Line wraps marked » —Ed.)
for (prop in attributes) { if (el.getAttribute(prop) != attributes[prop]) includeThisElement» = false;
It now stops just under the road that begins with for (prop
. Right here, you may see that the prop
variable is used with out being outlined as an area variable, with a var
key phrase contained in the operate. Merely altering it to for (var prop
will repair our third bug.
Bug 4: the clone attribute that shouldn’t be there#section11
The fourth bug was clearly discovered by an intensive tester utilizing a DOM inspector, as a result of it’s not seen from the consumer interface of the appliance. However, as quickly as you open the DOM inspectors (in Firebug it’s the “HTML” tab, whereas in Dragonfly the function is named “DOM”) it turns into clear that many parts have clone
attributes with JavaScript supply code in them that shouldn’t be there:
fig. 8: Dragonfly’s DOM inspector reveals the problematic code.
Each aspect created by the script has a superfluous clone
attribute. Because it’s one thing the tip consumer won’t ever discover from the consumer interface, it could not look like a severe bug. However think about the efficiency impression if this script is used to create a DOM tree with a whole lot or hundreds of parts…
The quickest solution to discover this drawback is to set a breakpoint that solely happens when an attribute named clone
is about on any HTML aspect. Can our debuggers deal with that?
JavaScript is a really versatile language, and certainly one of its strengths (or quirks, relying in your standpoint) is you can exchange core capabilities with your individual. Add this snippet of debugging code to the web page, and it’ll exchange the unique setAttribute()
technique with one which breaks if a clone
attribute is about:
var funcSetAttr = Factor.prototype.setAttribute; /* maintain a pointer
to the unique operate */Factor.prototype.setAttribute = operate(identify, worth) {
if (identify == 'clone') {
debugger; /* break if script units the 'clone' attribute */
}
funcSetAttr.name(this,identify,worth); /* name authentic operate to
guarantee these different attributes are set appropriately */
};
To seek out the clone
attribute creation level:
- Add the debugging code on the high of the primary script within the doc.
- Reload the web page.
As quickly as you reload the web page, the script begins producing the DOM, however breaks the primary time it makes an attempt to set the unhealthy attribute. (Notice that in present variations of Firefox, setAttribute
has numerous element-specific implementations. The code above solely works as anticipated in Opera; to get the identical impact in Firefox you may exchange the phrase Factor
with HTMLFormElement
to exchange the extra particular HTMLFormElement.prototype.setAttribute
technique.)
When execution stops on the breakpoint, you’ll wish to know the place the setAttribute()
name occurred. This implies it’s important to return up the record of referred to as capabilities and determine how the script obtained right here. For this, you’ll use the decision stack.
Determine 9 reveals Dragonfly’s and IE8’s name stacks when stopped on the breakpoint. Only for reference, I’ve created a guide breakpoint in IE8 at roughly the identical place the setAttribute()
approach stops. You may see that in Dragonfly essentially the most just lately referred to as operate is on the high—it’s the nameless operate that was mapped to setAttribute
. It was referred to as from makeElement
at line 95:
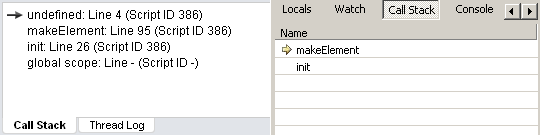
fig. 9: Dragonfly and IE8’s name stacks.
Determine 10 reveals Firebug’s name stack view. The setAttribute < makeElement < init
line subsequent to the file identify is the stack—and you may see that essentially the most just lately referred to as operate is the leftmost one:

fig. 10: The model of Firebug we used reveals the callstack on one line. The final operate referred to as is on the left.
By clicking earlier capabilities within the stack record you may step again via the calls and take a look at the way you arrived right here. It’s essential to do that your self to totally perceive how highly effective this function is. Notice that once you soar to a different stack entry, the native variable pane is up to date to indicate you the present state of the variables within the calling operate.
The right way to use the decision stack to seek out the offending operate:
- Click on the operate you wish to see within the name stack.
- Notice that the native variables pane updates to indicate the variables native to that operate.
- Keep in mind that should you use the step buttons, they may take you onwards from the final name even in case you are inspecting different elements of the stack.
Clicking the makeElement
reference takes us again to this half:
for (var prop in attributes) {
el.setAttribute(prop, attributes[prop]);
}
the place you see the setAttribute
name. The native variables/body inspection panes present that the worth of prop
is certainly clone
. The variable prop
is outlined within the for…in loop
. This tells us that one of many properties of the attributes
object should be named clone
.
The attributes
variable is outlined because the second named argument of that operate. For those who go one step as much as the init
a part of the stack, you may see that the earlier operate name was this:
var kind = makeElement(‘kind’, { motion:’/login’, technique:’publish’, identify:’loginform’ }, doc.physique);
The second argument to the tactic is highlighted in daring—this object doesn’t have a clone property. So the place does it come from?
For those who click on the makeElement
operate within the stack once more, you may examine the attributes
variable. In Firebug you’ll see the clone
property seem. You may click on the operate it references to leap to the definition—observe the road highlighted in blue in Determine 11:
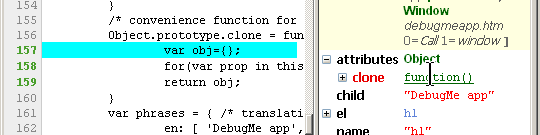
fig. 11: Firebug reveals us the place the clone property was outlined.
The Dragonfly alpha doesn’t embrace properties of an object’s prototype within the variable inspection view, so clone
doesn’t seem there. However now you’ve discovered {that a} clone
operate exists on all objects. For those who allow the “cease at new script” setting, and create a brand new thread calling this technique, you’ll soar proper to it.
To seek out the clone
definition in Dragonfly:
- Toggle the “cease at new script” choice with the consumer interface button proven on this screenshot:
- Paste the next code into the deal with bar of the window you might be debugging:
javascript:void({}.clone());
With a bookmarklet, you can begin new threads within the debugged doc at will. For instance, you possibly can strive javascript:void({}.clone());
to start out a brand new thread that can name the clone()
technique. When Dragonfly stops on the new thread, one click on on the “step into” button jumps to the problematic definition of the clone
property. (Notice {that a} Dragonfly bug could require disabling Consumer JavaScript to get this working.)
And right here’s the reason for the fourth bug: a clone technique is added to all objects utilizing Object.prototype
syntax. That is thought-about a nasty follow, as a result of utilizing for…in
on objects will see all the things you add to Object.prototype
. This may increasingly create bugs which are very onerous to trace down.
To repair this bug, you possibly can transfer the clone
technique in order that it’s outlined instantly on the Object
object as an alternative of on the prototype, after which repair any calls to obj.clone()
in order that they turn out to be Object.clone(obj)
as an alternative. For instance, keep away from this:
// BAD, do not do that:
Object.prototype.clone = operate() {
var obj = {};
for (var prop on this) {
obj[prop] = this[prop];
}
return obj;
}// some code that demonstrates utilizing the clone() technique:
var myObj1 = { 'id': '1' };
var myObj2 = myObj1.clone();
Do that as an alternative:
Object.clone = operate(originalObject) {
var obj = {};
for (var prop in originalObject) {
obj[prop] = originalObject[prop];
}
return obj;
}// some code that demonstrates utilizing the clone() technique:
var myObj1 = { 'id': '1' };
var myObj2 = Object.clone(myObj1);
The latter instance avoids modifying Object.prototype
and thus works higher with different scripts utilizing for…in
kind loops.
Bugs 5 via seven#section12
Now, pricey reader, you might be by yourself. Utilizing your new expertise, bugs 5, six, and 7 are as much as you to repair! Good luck and have enjoyable.
Abstract#section13
This text demonstrates the fundamentals of utilizing debuggers and a few superior JavaScript debugging strategies. You’ve discovered how you can set break factors each from the debugger and from scripts, how you can step via code, how you can use the debugger consumer interface, how you can set superior break factors, and how you can combine bookmarklets in your debugging strategies.
For those who had issues following the extra superior elements of this text, don’t fear! Mastering the fundamentals first will make you a significantly better developer and finally, you’ll develop your individual set of fascinating strategies to share.