Seventy-one p.c of as we speak’s web customers don’t communicate English as a primary language, and that quantity retains rising. However few folks focus on internationalization. In consequence, most websites get it improper—as a result of issues that appear easy are sometimes something however.
Article Continues Beneath
Take pluralization. Turning singular phrases into plurals inside strings will get tough rapidly—even in English, the place most plural phrases finish with an s. For example, I labored on a photo-sharing app that supported two languages, English and Chinese language. It was simple so as to add an s to show “X like[s]” or “Y remark[s].” However what if we would have liked to pluralize “foot” or “inch” or “quiz”? Our easy resolution turned a damaged hack.
And English is a comparatively easy case. Many languages have greater than two plural types: Arabic, for instance, has six, and lots of Slavic languages have greater than three. In truth, at the very least 39 languages have greater than two plural types. Some languages solely have one kind, reminiscent of Chinese language and Japanese, which means that plural and singular nouns are the identical.
How can we make sense of those advanced pluralization points—and resolve them in our tasks? On this article, I’ll present you a few of the most typical pluralization issues, and clarify find out how to overcome them.
Issues with pluralization#section2
Pluralization will get much more advanced: every language additionally has its personal guidelines for outlining every plural kind. A plural rule defines a plural kind utilizing a formulation that features a counter. A counter is the variety of objects you’re attempting to pluralize. Say we’re working with “2 rabbits.” The quantity earlier than the phrase “rabbits” is the counter. On this case, it has the worth 2. Now, if we take the English language for instance, it has two plural types: singular and plural. Due to this fact, our guidelines appear like this:
- If the counter has the integer worth of 1, use the singular: “rabbit.”
- If the counter has a price that’s not equal to 1, use the plural: “rabbits.”
Nevertheless, the identical isn’t true in Polish, the place the identical phrase—“rabbit,” or “królik”—can take greater than two types:
- If the counter has the integer worth of 1, use “królik.”
- If the counter has a price that ends in 2–4, excluding 12–14, use “królika.”
- If the counter just isn’t 1 and has a price that ends in both 0 or 1, or the counter ends in 5–9, or the counter ends in 12–14, use “królików.”
- If the counter has another worth than the above, use “króliki.”
A lot for “singular” and “plural.” For languages with three or extra plural types, we want extra particular labels.
Completely different languages use various kinds of numbers#section3
You may additionally need to show the counter together with the pluralized noun, reminiscent of, “You’ve gotten 3 rabbits.” Nevertheless, not all languages use the Arabic numbers you might be accustomed to—for instance, Arabic makes use of Arabic Indic numbers, ٠١٢٣٤٥٦٧٨٩:
- 0 books: ٠ كتاب
- 1 e book: كتاب
- 3 books: ٣ كتب
- 11 books: ١١ كتابًا
- 100 books: ١٠٠ كتاب
Completely different languages or areas use totally different quantity codecs#section4
We additionally typically intention to make massive numbers extra readable by including separators, as after we render the quantity 1000 as “1,000” in English. However many languages and areas use totally different fractional and thousand separators. For instance, German renders the quantity 1000 as “1.000.” Different languages don’t group numbers by hundreds, however reasonably by tens of hundreds.
Resolution: ICU’s MessageFormat#section5
Pluralization is a posh downside to resolve—at the very least, if you wish to deal with all these edge instances. Lately, Worldwide Parts for Unicode (ICU) did exactly that with MessageFormat. ICU’s MessageFormat is a markup language particularly tailor-made to localization. It permits you to outline, in a declarative means, how nouns ought to be rendered in numerous plural types. It types all of the plural types and guidelines for you, and codecs numbers appropriately. Sadly, lots of you most likely haven’t heard of MessageFormat but, as a result of it’s largely utilized by individuals who work particularly with internationalization—recognized to insiders as i18n—and JavaScript has solely lately advanced to deal with it.
Let’s discuss the way it works.
Utilizing CLDR for plural types#section6
CLDR stands for Frequent Locale Information Repository, and it’s a repo that corporations like Google, IBM, and Apple draw on to get details about quantity, date, and time formatting. CLDR additionally accommodates knowledge on the plural types and guidelines for a lot of languages. It’s most likely the biggest locale knowledge repository on the planet, which makes it splendid as the premise for any internationalization JavaScript device.
CLDR defines as much as six totally different plural types. Every kind is assigned a reputation: zero, one, two, few, many, or different. Not all locales want each kind; keep in mind, English solely has two: one and different. The title of every kind is predicated on its corresponding plural rule. Here’s a CLDR instance for the Polish language—a barely altered model of our earlier counter guidelines:
- If the counter has the integer worth of 1, use the plural kind one.
- If the counter has a price that ends in 2–4, excluding 12–14, use the plural kind few.
- If the counter just isn’t 1 and has a price that ends in both 0 or 1, or the counter ends in 5–9, or the counter ends in 12–14, use the plural kind many.
- If the counter has another worth than the above, use the plural kind different.
As an alternative of manually implementing CLDR plural types, you can also make use of instruments and libraries. For instance, I created L10ns, which compiles the code for you; Yahoo’s FormatJS has all of the plural types in-built. The massive advantages of those instruments and libraries are that they scale nicely, as they summary the plural-form dealing with. Should you select to hard-code these plural types your self, you’ll find yourself exhausting your self and your teammates, since you’ll must preserve monitor of all of the types and guidelines, and outline them time and again at any time when and wherever you need to format a plural string.
MessageFormat#section7
MessageFormat is a domain-specific language that makes use of CLDR, and is particularly tailor-made for localizing strings. You outline markup inline. For instance, we need to format the message “I’ve X rabbit[s]” utilizing the proper plural phrase for “rabbit”:
var message="I've {rabbits, plural, one{# rabbit} different{# rabbits}}";
As you may see, a plural format is outlined inside curly brackets {}
. It takes a counter, rabbits
, as the primary argument. The second argument defines which kind of formatting. The third argument consists of CLDR’s plural kind (one
, many
). It is advisable outline a sub-message contained in the curly brackets that corresponds to every plural kind. You may also move within the image #
to render the counter with the right quantity format and numbering system, so it should resolve the issues we recognized earlier with the Arabic Indic numbering system and with quantity formatting.
Right here we parse the message within the en-US locale and output totally different messages relying on which plural kind the variable rabbits
takes:
var message="I've {rabbits, plural, one{# rabbit} different{# rabbits}}.";
var messageFormat = new MessageFormat('en-US');
var output = messageFormat.parse(message);
// Will output "I've 1 rabbit."
console.log(output({ rabbits: 1 }));
// Will output "I've 10 rabbits."
console.log(output({ rabbits: 10 }));
Advantages of inlining#section8
As you may see within the previous message, we outlined a plural format inline. If it weren’t inlined, we’d must repeat the phrases “I’ve…” for all plural types, as a substitute of simply typing them as soon as. Think about for those who wanted to make use of much more phrases, as within the following instance:
{
one: 'My title is Emily and I obtained 1 like in my newest put up.'
different: 'My title is Emily and I obtained # likes in my newest put up.'
}
With out inlining, we’d must repeat “My title is Emily and I obtained…in my newest put up” each single time. That’s quite a lot of phrases.
In distinction, inlining in ICU’s MessageFormat simplifies issues. As an alternative of repeating the phrase for each plural kind, all we have to do is localize the phrase “like”:
var message="My title is Emily and I obtained {likes, plural, one{# like} different{# likes}} in my newest put up";
Right here we don’t must repeat the phrases “My title is Emily and I obtained…in my newest put up” for each plural kind. As an alternative, we are able to merely localize the phrase “like.”
Advantages of nesting messages#section9
MessageFormat’s nested nature additionally helps us by giving us infinite prospects to outline a large number of advanced strings. Right here we outline a choose format in a plural format to reveal how versatile MessageFormat is:
var message="{likeRange, choose,
range1{I obtained no likes}
range2{I obtained {likes, plural, one{# like} different{# likes}}}
different{I obtained too many likes}
}";
A choose format matches a set of instances and, relying on which case it matches, it outputs the corresponding sub-message. And it’s excellent to assemble range-based messages. Within the previous instance, we need to assemble three sorts of messages for every like
vary. As you may see in range2
, we outlined a plural format to format the message “I obtained X like[s],” after which nested the plural format inside a choose format. This instance showcases a really advanced formatting that only a few syntaxes can obtain, demonstrating MessageFormat’s flexibility.
With the above format, listed below are the messages we are able to count on to get:
- “I obtained no likes,” if
likeRange
is inrange1
. - “I obtained 1 like,” if
likeRange
is inrange2
and the variety of likes is 1. - “I obtained 10 likes,” if
likeRange
is inrange2
and the variety of likes is 10. - “I obtained too many likes,” if
likeRange
is in neitherrange1
norrange2
.
These are very laborious ideas to localize—even one of the crucial standard internationalization instruments, gettext, can’t do that.
Storage and pre-compiled messages#section10
Nevertheless, as a substitute of storing MessageFormat messages in a JavaScript variable, you may need to use some sort of storage format, reminiscent of a number of JSON recordsdata. This can help you pre-compile the messages to easy localization getters. Should you don’t need to deal with this alone, you may strive L10ns, which handles storage and pre-compilation for you, in addition to syncing translation keys between supply and storage.
Do translators must know MessageFormat?#section11
You may suppose it could be too overwhelming for non-programming translators to know Messageformat and CLDR’s plural kind. However in my expertise, educating them the fundamentals of how the markup appears and what it does, and what CLDR’s plural types are, takes only a few minutes and gives sufficient info for translators to do their job utilizing MessageFormat. L10ns’ net interface additionally shows the instance numbers for every CLDR plural kind for straightforward reference.
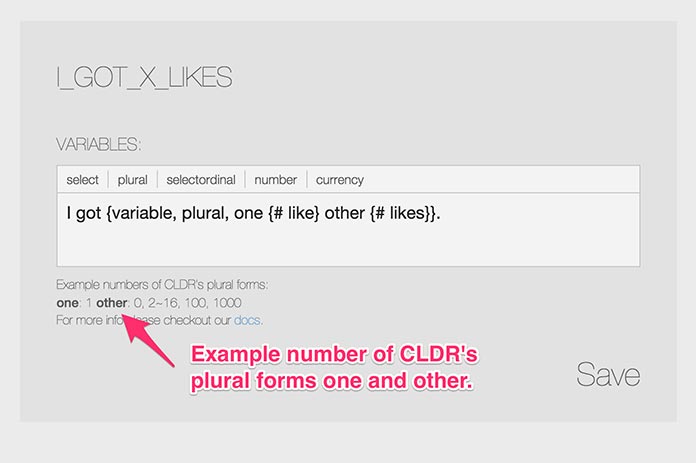
Pluralization isn’t simple—but it surely’s value it#section12
Sure, pluralization has quite a lot of edge instances that aren’t simply solvable. However ICU’s MessageFormat has helped me tremendously in my work, giving me infinite flexibility to translate plural strings. As we transfer to a extra related world, localizing functions to extra languages and areas is a must-do. Information about common localization issues and instruments to resolve them are must-haves. We have to localize apps as a result of the world is extra related, however we are able to additionally localize apps to assist make the world extra related.