By the numbers, JavaScript is a efficiency legal responsibility. If the pattern persists, the median web page shall be transport no less than 400 KB of it earlier than too lengthy, and that’s merely what’s transferred. Like different text-based assets, JavaScript is nearly all the time served compressed—however that is likely to be the one factor we’re getting persistently proper in its supply.
Article Continues Under
Sadly, whereas decreasing useful resource switch time is an enormous a part of that entire efficiency factor, compression has no impact on how lengthy browsers take to course of a script as soon as it arrives in its entirety. If a server sends 400 KB of compressed JavaScript, the precise quantity browsers need to course of after decompression is north of a megabyte. How effectively units deal with these heavy workloads relies upon, effectively, on the gadget. A lot has been written about how adept varied units are at processing plenty of JavaScript, however the reality is, the period of time it takes to course of even a trivial quantity of it varies enormously between units.
Take, for instance, this throwaway undertaking of mine, which serves round 23 KB of uncompressed JavaScript. On a mid-2017 MacBook Professional, Chrome chews by this comparably tiny payload in about 25 ms. On a Nokia 2 Android telephone, nonetheless, that determine balloons to round 190 ms. That’s not an insignificant period of time, however in both case, the web page will get interactive fairly quick.
Now for the massive query: how do you suppose that little Nokia 2 does on a median web page? It chokes. Even on a quick connection, looking the net on it’s an train in endurance as JavaScript-laden net pages brick it for appreciable stretches of time.

Whereas units and the networks they navigate the net on are largely bettering, we’re consuming these features as traits counsel. We have to use JavaScript responsibly. That begins with understanding what we’re constructing in addition to how we’re constructing it.
The mindset of “websites” versus “apps”#section2
Nomenclature might be unusual in that we generally loosely establish issues with phrases which are inaccurate, but their meanings are implicitly understood by everybody. Typically we overload the time period “bee” to additionally imply “wasp”, despite the fact that the variations between bees and wasps are substantial. These variations can encourage you to cope with every one in another way. For example, we’ll wish to destroy a wasp nest, however as a result of bees are extremely helpful and weak bugs, we might decide to relocate them.
We might be simply as quick and unfastened in interchanging the phrases “web site” and “net app”. The variations between them are much less clear than these between yellowjackets and honeybees, however conflating them can result in painful outcomes. The ache comes within the affordances we enable ourselves when one thing is merely a “netwebsite” versus a fully-featured “net app.” Should you’re making an informational web site for a enterprise, you’re much less prone to lean on a robust framework to handle modifications within the DOM or implement client-side routing—no less than, I hope. Utilizing instruments so ill-suited for the duty wouldn’t solely be a detriment to the individuals who use that website however arguably much less productive.
Once we construct an online app, although, look out. We’re putting in packages which usher in lots of—if not hundreds—of dependencies, a few of which we’re unsure are even protected. We’re additionally writing sophisticated configurations for module bundlers. On this frenzied, but ubiquitous, kind of dev setting, it takes information and vigilance to make sure what will get constructed is quick and accessible. Should you doubt this, run npm ls --prod
in your undertaking’s root listing and see in case you acknowledge all the pieces in that record. Even in case you do, that doesn’t trương mục for third social gathering scripts—of which I’m positive your website has no less than a number of.
What we are likely to neglect is that the setting web sites and net apps occupy is one and the identical. Each are topic to the identical environmental pressures that the massive gradient of networks and units impose. These constraints don’t immediately vanish once we resolve to name what we construct “apps”, nor do our customers’ telephones achieve magical new powers once we accomplish that.
It’s our accountability to guage who makes use of what we make, and settle for that the circumstances underneath which they entry the web might be completely different than what we’ve assumed. We have to know the aim we’re attempting to serve, and solely then can we construct one thing that admirably serves that goal—even when it isn’t thrilling to construct.
Meaning reassessing our reliance on JavaScript and the way the usage of it—notably to the exclusion of HTML and CSS—can tempt us to undertake unsustainable patterns which hurt efficiency and accessibility.
Don’t let frameworks pressure you into unsustainable patterns#section3
I’ve been witness to some unusual discoveries in codebases when working with groups that depend upon frameworks to assist them be extremely productive. One attribute widespread amongst a lot of them is that poor accessibility and efficiency patterns typically end result. Take the React part beneath, for instance:
import React, { Element } from "react";
import { validateEmail } from "helpers/validation";
class SignupForm extends Element {
constructor (props) {
tremendous(props);
this.handleSubmit = this.handleSubmit.bind(this);
this.updateEmail = this.updateEmail.bind(this);
this.state.e-mail = "";
}
updateEmail (occasion) {
this.setState({
e-mail: occasion.goal.worth
});
}
handleSubmit () {
// If the e-mail checks out, submit
if (validateEmail(this.state.e-mail)) {
// ...
}
}
render () {
return (
Enter your e-mail:
);
}
}
There are some notable accessibility points right here:
- A kind that doesn’t use a
<kind>
component is not a kind. Certainly, you would paper over this by specifyingposition="kind"
within the dad or mum<div>
, however in case you’re constructing a kind—and this positive appears to be like like one—use a<kind>
component with the correctmotion
andmethodology
attributes. Themotion
attribute is essential, because it ensures the shape will nonetheless do one thing within the absence of JavaScript—supplied the part is server-rendered, after all. - A
<span>
isn’t an alternative choice to a<label>
component, which offers accessibility advantages<span>
s don’t. - If we intend to do one thing on the consumer facet previous to submitting a kind, then we should always transfer the motion sure to the
<button>
component’sonClick
handler to the<kind>
component’sonSubmit
handler. - By the way, why use JavaScript to validate an e-mail handle when HTML5 gives kind validation controls in virtually each browser again to IE 10? There’s a possibility right here to depend on the browser and use an applicable enter sort, in addition to the
required
attribute—however remember that getting this to work proper with display screen readers takes somewhat know-how. - Whereas not an accessibility challenge, this part doesn’t depend on any state or lifecycle strategies, which implies it may be refactored right into a stateless practical part, which makes use of significantly much less JavaScript than a full-fledged React part.
Understanding these items, we are able to refactor this part:
import React from "react";
const SignupForm = props => {
const handleSubmit = occasion => {
// Wanted in case we're sending knowledge to the server XHR-style
// (however will nonetheless work if server-rendered with JS disabled).
occasion.preventDefault();
// Stick with it...
};
return (
<kind methodology="POST" motion="/signup" onSubmit={handleSubmit}>
<label for="e-mail" class="email-label">Enter your e-mail:</label>
<enter sort="e-mail" id="e-mail" required />
<button>Signal Up</button>
</kind>
);
};
Not solely is that this part now extra accessible, however it additionally makes use of much less JavaScript. In a world that’s drowning in JavaScript, deleting strains of it ought to really feel downright therapeutic. The browser provides us a lot totally free, and we should always attempt to reap the benefits of that as typically as doable.
This isn’t to say that inaccessible patterns happen solely when frameworks are used, however quite {that a} sole desire for JavaScript will ultimately floor gaps in our understanding of HTML and CSS. These information gaps will typically lead to errors we might not even concentrate on. Frameworks might be helpful instruments that enhance our productiveness, however persevering with schooling in core net applied sciences is important to creating usable experiences, it doesn’t matter what instruments we select to make use of.
Rely on the internet platform and also you’ll go far, quick#section4
Whereas we’re as regards to frameworks, it have to be mentioned that the net platform is a formidable framework of its personal. Because the earlier part confirmed, we’re higher off once we can depend on established markup patterns and browser options. The choice is to reinvent them, and invite all of the ache such endeavors all however assure us, or worse: merely assume that the writer of each JavaScript package deal we set up has solved the issue comprehensively and thoughtfully.
SINGLE PAGE APPLICATIONS#section5
One of many tradeoffs builders are fast to make is to undertake the only web page software (SPA) mannequin, even when it’s not a match for the undertaking. Sure, you do achieve higher perceived efficiency with the client-side routing of an SPA, however what do you lose? The browser’s personal navigation performance—albeit synchronous—offers a slew of advantages. For one, historical past is managed in keeping with a fancy specification. Customers with out JavaScript—be it by their very own selection or not—received’t lose entry altogether. For SPAs to stay obtainable when JavaScript isn’t, server-side rendering immediately turns into a factor it’s a must to contemplate.
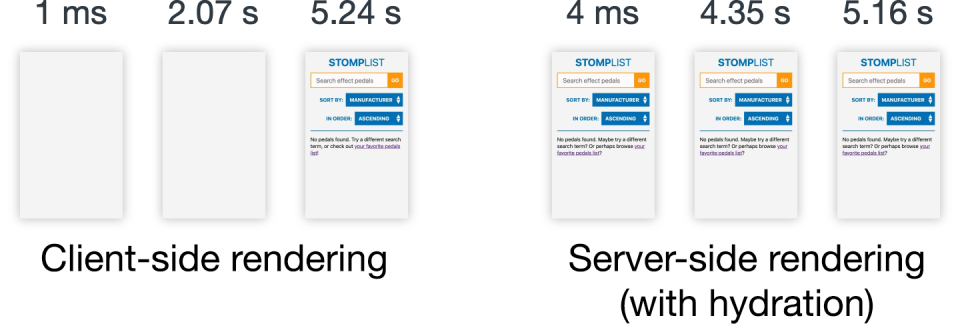
Accessibility can also be harmed if a client-side router fails to let folks know what content material on the web page has modified. This may go away these reliant on assistive know-how to suss out what modifications have occurred on the web page, which might be an arduous process.
Then there’s our outdated nemesis: overhead. Some client-side routers are very small, however once you begin with React, a suitable router, and presumably even a state administration library, you’re accepting that there’s a specific amount of code you may by no means optimize away—roughly 135 KB on this case. Fastidiously contemplate what you’re constructing and whether or not a consumer facet router is definitely worth the tradeoffs you’ll inevitably make. Sometimes, you’re higher off with out one.
Should you’re involved concerning the perceived navigation efficiency, you might lean on rel=prefetch
to speculatively fetch paperwork on the identical origin. This has a dramatic impact on bettering perceived loading efficiency of pages, because the doc is instantly obtainable within the cache. As a result of prefetches are achieved at a low precedence, they’re additionally much less prone to take care of crucial assets for bandwidth.
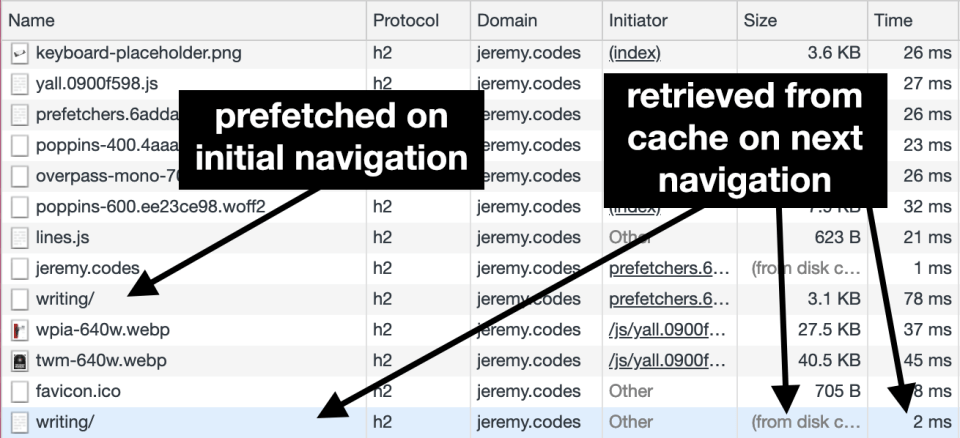
The first downside with hyperlink prefetching is that it’s essential remember that it can be doubtlessly wasteful. Quicklink, a tiny hyperlink prefetching script from Google, mitigates this considerably by checking if the present consumer is on a gradual connection—or has knowledge saver mode enabled—and avoids prefetching hyperlinks on cross-origins by default.
Service employees are additionally vastly helpful to perceived efficiency for returning customers, whether or not we use consumer facet routing or not—supplied you already know the ropes. Once we precache routes with a service employee, we get lots of the identical advantages as hyperlink prefetching, however with a a lot better diploma of management over requests and responses. Whether or not you consider your website as an “app” or not, including a service employee to it’s maybe probably the most accountable makes use of of JavaScript that exists right now.
JAVASCRIPT ISN’T THE SOLUTION TO YOUR LAYOUT WOES#section6
If we’re putting in a package deal to unravel a format drawback, proceed with warning and ask “what am I attempting to perform?” CSS is designed to do that job, and requires no abstractions to make use of successfully. Most format points JavaScript packages try to unravel, like field placement, alignment, and sizing, managing textual content overflow, and even total format techniques, are solvable with CSS right now. Trendy format engines like Flexbox and Grid are supported effectively sufficient that we shouldn’t want to start out a undertaking with any format framework. CSS is the framework. When we have now characteristic queries, progressively enhancing layouts to undertake new format engines is immediately not so laborious.
/* Your mobile-first, non-CSS grid kinds goes right here */
/* The @helps rule beneath is ignored by browsers that do not
help CSS grid, _or_ do not help @helps. */
@helps (show: grid) {
/* Bigger display screen format */
@media (min-width: 40em) {
/* Your progressively enhanced grid format kinds go right here */
}
}
Utilizing JavaScript options for format and displays issues isn’t new. It was one thing we did once we lied to ourselves in 2009 that each web site needed to look in IE6 precisely because it did within the extra succesful browsers of that point. If we’re nonetheless growing web sites to look the identical in each browser in 2019, we should always reassess our improvement objectives. There’ll all the time be some browser we’ll need to help that may’t do all the pieces these fashionable, evergreen browsers can. Whole visible parity on all platforms isn’t solely a pursuit made in useless, it’s the principal foe of progressive enhancement.
I’m not right here to kill JavaScript#section7
Make no mistake, I’ve no unwell will towards JavaScript. It’s given me a profession and—if I’m being trustworthy with myself—a supply of enjoyment for over a decade. Like all long-term relationship, I study extra about it the extra time I spend with it. It’s a mature, feature-rich language that solely will get extra succesful and chic with each passing yr.
But, there are occasions after I really feel like JavaScript and I are at odds. I am crucial of JavaScript. Or possibly extra precisely, I’m crucial of how we’ve developed an inclination to view it as a primary resort to constructing for the net. As I decide aside yet one more bundle not in contrast to a tangled ball of Christmas tree lights, it’s change into clear that the net is drunk on JavaScript. We attain for it for nearly all the pieces, even when the event doesn’t name for it. Typically I’m wondering how vicious the hangover shall be.
In a collection of articles to observe, I’ll be giving extra sensible recommendation to observe to stem the encroaching tide of extreme JavaScript and the way we are able to wrangle it in order that what we construct for the net is usable—or no less than extra so—for everybody in every single place. A number of the recommendation shall be preventative. Some shall be mitigating “hair of the canine” measures. In both case, the outcomes will hopefully be the identical. I consider that all of us love the net and wish to do proper by it, however I would like us to consider tips on how to make it extra resilient and inclusive for all.