One other technology of know-how has handed and Unicode assist is nearly in all places. The following step is to put in writing software program that isn’t simply “internationalized” however really multilingual. On this article we are going to skip by means of a little bit of historical past and principle, then illustrate a neat hack known as accent-folding. Accent-folding has its limitations however it will possibly assist make some essential but ignored person interactions work higher.
Article Continues Beneath
A standard assumption about internationalization is that each person matches right into a single locale like “English, United States” or “French, France.” It’s a hangover from the PC days when simply getting the pc to show the proper squiggly bits was a giant deal. One byte equaled one character, no exceptions, and you may solely load one language’s alphabet at a time. This was positive as a result of it was higher than nothing, and since customers spent most of their time with paperwork they or their coworkers produced themselves.
As we speak customers cope with information from in all places, in a number of languages and locales, on a regular basis. The locale I desire is simply loosely correlated with the locales I count on functions to course of.
Take into account this deal with e-book:
- Fulanito López
- Erik Lørgensen
- Lorena Smith
- James Lö
If I compose a brand new message and kind “lo” within the To: discipline, what ought to occur? In lots of functions solely Lorena will present up. These functions “assist Unicode,” within the sense that they don’t corrupt or barf on it, however that’s all.
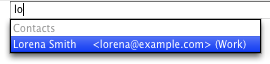
Fig 1. Hey Entourage, the place are my contacts?
This downside is not only in deal with books. Take into consideration inboxes, social bookmarks, remark feeds, customers who communicate a number of languages, customers in web cafés in international international locations, even URLs. Have a look at the journalist Ryszard Kapuściński and the way totally different web sites deal with his identify:
There isn’t a excuse on your software program to play dumb when the person sorts “cafe” as a substitute of “café.”
Áçčềñṭ-Ḟøłɖǐṅg#section2
In particular functions of search that favor recall over precision, equivalent to our deal with e-book instance, á, a, å, and â could be handled as equal. Accents (a.okay.a diacritical marks) are pronunciation hints that don’t have an effect on the textual that means. Getting into them could be cumbersome, particularly on cellular gadgets.
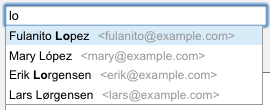
Fig 2. accent-folding in an autosuggest widget
An accent-folding operate basically maps Unicode characters to ASCII equivalents. Anyplace you apply case-folding, it’s best to think about accent-folding, and for precisely the identical causes. With accent-folding, it doesn’t matter whether or not customers seek for cafe, café and even çåFé; the outcomes would be the similar.
Bear in mind that there are 1,000,000 caveats to accent guidelines. You’ll virtually actually get it fallacious for anyone, someplace. Almost each alphabet has a couple of extra-special marks that do have an effect on that means, and, in fact, non-Western alphabets have utterly totally different guidelines.
A minor gotcha is the Unicode “fullwidth” Roman alphabet. These are fixed-width variations of plain ASCII characters designed to line up with Chinese language/Japanese/Korean characters (e.g., “1979年8月15日”). They reside in 0xff00
to 0xff5e
and needs to be handled as equal to their ASCII counterparts.
Hey man, I’m solely right here for the copy/paste#section3
I’ve posted extra full examples on GitHub, however for illustration, right here’s a fundamental accent-folder in Javascript:
(Line wraps marked » —Ed.)
var accentMap = {
'á':'a', 'é':'e', 'í':'i','ó':'o','ú':'u'
};operate accent_fold (s) {
if (!s) { return ''; }
var ret="";
for (var i = 0; i < s.size; i++)
return ret;
};
Common Expressions#section4
Common expressions are very tough to make accent-aware. Discover that in Fig. 2 solely the unaccented entries are in daring kind. The issue is that the Unicode character structure doesn’t lend itself to patterns that reduce throughout languages. The correct regex for “lo” can be one thing insane like:
[LlĹ弾ĻļḶḷḸḹḼḽḺḻŁłŁłĿŀȽƚɫ][OoÓóÒòŎŏÔôỐốỒồỖöȪȫŐőÕõṌȭȮȯǾǿ...ǬǭŌ]
By no means, by no means do that. As of this writing, few common expression engines assist shortcuts for Unicode character courses. PCRE and Java appear to be within the vanguard. You most likely shouldn’t push it. As an alternative, strive highlighting an accent-folded model of the string, after which use these character positions to spotlight the unique, like so:
<b>// accent_folded_hilite("Fulanilo López", 'lo')
// --> "Fulani<b>lo</b> <b>Ló</b>pez"
//
operate accent_folded_hilite(str, q) {
var str_folded = accent_fold(str).toLowerCase() »
.change(/[<>]+/g, '');
var q_folded = accent_fold(q).toLowerCase() »
.change(/[<>]+/g, ''); // Create an intermediate string with hilite hints
// Instance: fulani<lo> <lo>pez
var re = new RegExp(q_folded, 'g');
var hilite_hints = str_folded.change(re, »
'<'+q_folded+'>'); // Index pointer for the unique string
var spos = 0;
// Accumulator for our last string
var highlighted = ''; // Stroll down the unique string and the hilite trace
// string in parallel. Whenever you encounter a < or > trace,
// append the opening / closing tag in our last string.
// If the present char is just not a touch, append the equiv.
// char from the unique string to our last string and
// advance the unique string's pointer.
for (var i = 0; i< hilite_hints.size; i++) {
var c = str.charAt(spos);
var h = hilite_hints.charAt(i);
if (h === '<') {
highlighted += '<b>';
} else if (h === '>') {
highlighted += '</b>';
} else {
spos += 1;
highlighted += c;
}
}
return highlighted;
}
The earlier instance might be too simplistic for manufacturing code. You may’t spotlight a number of phrases, for instance. Some particular characters may develop to 2 characters, equivalent to “æ” –> “ae” which is able to screw up spos
. It additionally strips out angle-brackets (<>) within the unique string. But it surely’s ok for a primary go.
Accent-folding in YUI Autocomplete#section5
YUI’s Autocomplete library has many hooks and choices to play with. As we speak we’ll have a look at two overrideable strategies: filterResults()
and formatMatch()
. The filterResults
methodology permits you to write your personal matching operate. The formatMatch
methodology permits you to change the HTML of an entry within the checklist of recommended matches. It’s also possible to obtain an entire, working instance with all the supply code.
(Line wraps marked » —Ed.)
<b><!-- that is essential to inform javascript to deal with
the strings as UTF-8 -->
<meta http-equiv="content-type" content material="textual content/html;charset=utf-8" /><!-- YUI stylesheets -->
<hyperlink rel="stylesheet" kind="textual content/css"
href="http://yui.yahooapis.com/2.7.0/construct/fonts/fonts-min.css" />
<hyperlink rel="stylesheet" kind="textual content/css"
href="http://yui.yahooapis.com/2.7.0/construct/autocomplete/belongings »
/skins/sam/autocomplete.css" /><!-- YUI libraries: occasions, datasource and autocomplete -->
<script kind="textual content/javascript"
src="http://yui.yahooapis.com/2.7.0/construct/yahoo-dom-event »
/yahoo-dom-event.js"></script>
<script kind="textual content/javascript"
src="http://yui.yahooapis.com/2.7.0/construct/datasource »
/datasource-min.js"></script>
<script kind="textual content/javascript"
src="http://yui.yahooapis.com/2.7.0/construct/autocomplete »
/autocomplete-min.js"></script><!-- comprises accent_fold() and accent_folded_hilite() -->
<script kind="textual content/javascript" src="https://alistapart.com/article/accent-folding-for-auto-complete/accent-fold.js"></script><!-- Give <physique> the YUI "pores and skin" -->
<physique class="yui-skin-sam">
<b>To:</b>
<div model="width:25em"> <!-- Our to: discipline -->
<enter id="to" kind="textual content" /> <!-- An empty <div> to include the autocomplete -->
<div id="ac"></div> </div>
</physique><script>// Our static deal with e-book as a listing of hash tables
var addressBook = [
{name:'Fulanito López', email:'[email protected]'},
{name:'Erik Lørgensen', email:'[email protected]'},
{name:'Lorena Smith', email:'[email protected]'},
{name:'James Lö', email:'[email protected]'}
];/*
Iterate our deal with e-book and add a brand new discipline to every
row known as "search." This comprises an accent-folded
model of the "identify" discipline.
*/
for (var i = 0; i< addressBook.size; i++) {
addressBook[i]['search'] = accent_fold(addressBook[i]['name']);
}// Create a YUI datasource object from our uncooked deal with e-book
var datasource = new YAHOO.util.LocalDataSource(addressBook);/*
A datasource is tabular, however our array of hash tables has no
idea of column order. So explicitly inform the datasource
what order to place the columns in.
*/
datasource.responseSchema = {fields : ["email", "name", "search"]};/*
Instantiate the autocomplete widget with a reference to the
enter discipline, the empty div, and the datasource object.
*/
var autocomp = new YAHOO.widget.AutoComplete("to", "ac", datasource);// Enable a number of entries by specifying house
// and comma as delimiters
autocomp.delimChar = [","," "];/*
Add a brand new filterResults() methodology to the autocomplete object:
Iterate over the datasource and seek for q contained in the
"search" discipline. This methodology known as every time the person
sorts a brand new character into the enter discipline.
*/
autocomp.filterResults = operate(q, entries, resultObj, cb) {
var matches = [];
var re = new RegExp('b'+accent_fold(q), 'i');
for (var i = 0; i < entries.size; i++) {
if (re.check(entries[i]['search'])) {
matches.push(entries[i]);
}
}
resultObj.outcomes = matches;
return resultObj;
};/*
Add a brand new formatResult() methodology. It's known as on every end result
returned from filterResults(). It outputs a reasonably HTML
illustration of the match. On this methodology we run the
accent-folded spotlight operate over the identify and e mail.
*/
autocomp.formatResult = operate (entry, q, match) {
var identify = accent_folded_hilite(entry[1], q);
var e mail = accent_folded_hilite(entry[0], q);
return identify + ' <' + e mail + '>';
};//fin
</script>
About these million caveats…#section6
This accent-folding trick works primarily for Western European textual content, but it surely received’t work for all of it. It exploits particular quirks of the language household and the restricted downside domains of our examples, the place it’s higher to get extra outcomes than no outcomes. In German, Ü ought to most likely map to Ue as a substitute of simply U. A French particular person looking the online for thé (tea) can be upset if flooded with irrelevant English textual content.
You may solely push a easy character map to this point. It will be very tough to reconcile the Roman “Marc Chagall” with the Cyrillic “Марк Шагал” or Yiddish “מאַרק שאַגאַל.” There are very attention-grabbing similarities within the characters however a magical context-free two-way mapping might be not doable.
On prime of all that there’s one other downside: One language can have a couple of writing system. Transliteration is writing a language in a distinct alphabet. It’s not fairly the identical as transcription, which maps sounds as in “hola, que paso” –> “oh-la, keh pah-so.” Transliterations attempt to map the written symbols to a different alphabet, ideally in a method that’s reversible.
These 4 sentences all say “Kids like to observe tv” in Japanese:
- Kanji: 子供はテレビを見るのが好きです。
- Hiragana: こども は てれび を みる の が すき です 。
- Romaji: kodomo wa terebi o miru noga suki desu.
- Cyrillic: кодомо ва тэрэби о миру нога суки дэсу.
For hundreds of years folks have been inventing methods to put in writing totally different languages with no matter keyboards or typesetting they’d out there. So even when the person reads just one language, they may accomplish that in a number of transliteration schemes. Some schemes are logical and educational, however typically they’re messy natural issues that depend upon regional accent and historic cruft. The pc period kicked off a brand new explosion of techniques as folks realized to talk and ship emails in plain ASCII.
There’s a variety of prior work on this downside and two prepared paths you’ll be able to select: The correct method and the maybe-good-enough method. Neither have the simplicity of our naïve hash desk, however they are going to be much less disappointing on your customers generally functions.
The primary is Worldwide Parts for Unicode (ICU), a challenge that originated within the early nineties within the European Union. It goals to be an entire, language-aware transliteration, Unicode, formatting, every part library. It’s huge, it’s C++/Java, and it requires contextual information of the inputs and outputs to work.
The second is Unidecode, a context-free transliteration library out there for Perl and Python. It tries to transliterate all Unicode characters to a fundamental Latin alphabet. It makes little try to be reversible, language-specific, and even typically appropriate; it’s a quick-and-dirty hack that nonetheless is fairly complete.
Accent-folding in the proper locations saves your customers time and makes your software program smarter. The strict segregation of languages and locales is partially an artifact of know-how limitations which not maintain. It’s as much as you ways far you need to take issues, however even a little bit little bit of effort goes a great distance. Good luck!